EN
JavaScript - signal linear quantizer
10 points
In this short article, we would like to show how to create simple signal linear quantizer using JavaScript.
Quantization formula:
Where:
- Q(y) - output quantized value,
- y - input quantized value
- Δ - quantization step size
Quantization preview:
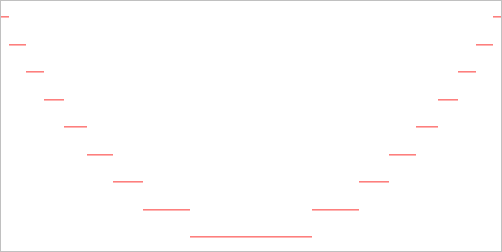
Practical example:
xxxxxxxxxx
1
const createLinearQuantizer = (step) => {
2
return (value) => {
3
return step * Math.floor(value / step + 0.5);
4
};
5
};
6
7
8
// Usage example:
9
10
const quantizer = createLinearQuantizer(10); // 10 is quantization step size
11
12
for (let x = -9; x <= 9; x += 0.3) {
13
const y = x * x; // typical parabola
14
const q = quantizer(y); // quantized parabola
15
console.log(`x=${x.toFixed(2)} Q(y)=${q}`);
16
}