EN
JavaScript - rotate localized vector 2D to left direction (counterclockwise direction)
7 points
In this short article, we would like to show how to rotate any localized vector 2D to the left side (90 degrees) using Javascript.
Quick solution:
xxxxxxxxxx
1
function rotateLeft(vector) {
2
var a = vector.a;
3
var b = vector.b;
4
return {
5
a: { x: -a.y, y: a.x }, // check: https://dirask.com/posts/1GoW61
6
b: { x: -b.y, y: b.x }
7
};
8
}
9
10
var vector1 = {a: {x: 1, y: 2}, b: {x: 2, y: 4}}; // vector [A1, B1] = ( 1, 2) -> ( 2, 4)
11
var vector2 = rotateLeft(vector1); // vector [A2, B2] = (-2, 1) -> (-4, 2)
Rotation preview:
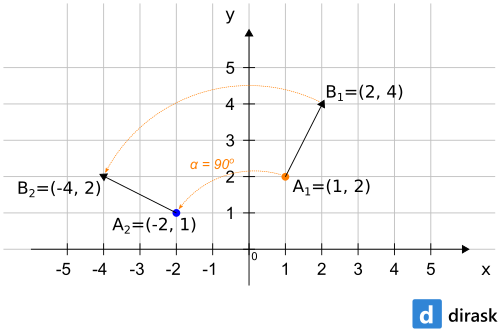
xxxxxxxxxx
1
function rotateLeft(vector) {
2
var a = vector.a;
3
var b = vector.b;
4
return {
5
a: { x: -a.y, y: a.x }, // check: https://dirask.com/posts/1GoW61
6
b: { x: -b.y, y: b.x }
7
};
8
}
9
10
11
// Helper methods:
12
13
function printPoint(point) {
14
return '(' + point.x + ', ' + point.y + ')';
15
}
16
17
function printVector(name, vector) {
18
console.log(name + ': ' + printPoint(vector.a) + ' -> ' + printPoint(vector.b));
19
}
20
21
22
// Usage example:
23
// direction:
24
var vector1 = { // ↑
25
a: {x: 0, y: 1}, // a = beginning point
26
b: {x: 0, y: 2} // b = ending point (arrow/head)
27
};
28
29
var vector2 = rotateLeft(vector1); // ←
30
var vector3 = rotateLeft(vector2); // ↓
31
var vector4 = rotateLeft(vector3); // →
32
33
printVector('vector1', vector1); // ( 0, 1) -> ( 0, 2) ↑
34
printVector('vector2', vector2); // (-1, 0) -> (-2, 0) ←
35
printVector('vector3', vector3); // ( 0, -1) -> ( 0, -2) ↓
36
printVector('vector4', vector4); // ( 1, 0) -> ( 2, 0) →