EN
JavaScript - rotate vector 2D to right direction (clockwise direction)
4 points
In this short article, we would like to show how to rotate any vector 2D to the right side (90 degrees) using Javascript.
Quick solution:
xxxxxxxxxx
1
function rotateRight(vector) {
2
return { x: vector.y, y: -vector.x };
3
}
4
5
var vector1 = {x: -2, y: 4}; // vector V1 = [-2, 4]
6
var vector2 = rotateRight(vector1); // vector V2 = [ 4, 2]
Rotation preview:
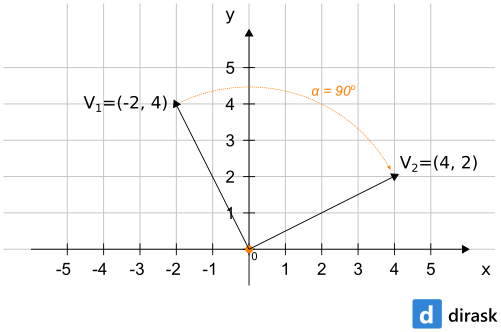
xxxxxxxxxx
1
function rotateRight(vector) {
2
return { x: vector.y, y: -vector.x };
3
}
4
5
6
// Usage example:
7
8
// direction:
9
var vector1 = {x: 0, y: 1}; // ↑
10
11
var vector2 = rotateRight(vector1); // →
12
var vector3 = rotateRight(vector2); // ↓
13
var vector4 = rotateRight(vector3); // ←
14
15
console.log(vector1.x + ' ' + vector1.y); // 0 1 ↑
16
console.log(vector2.x + ' ' + vector2.y); // 1 0 →
17
console.log(vector3.x + ' ' + vector3.y); // 0 -1 ↓
18
console.log(vector4.x + ' ' + vector4.y); // -1 0 ←
Note: the above example works on free vectors - typical mathematical vectors that are not localised.