EN
Java - convert SVG file to PNG file
7 points
In this article, we would like to show how to convert SVG file to PNG file using Java.
By default Java ImageIO
doesn't support SVG files. It is necessary to use some external implementation that is Apache™ Batik SVG Toolkit.
The article contains example project that let's to convert SVG files to PNG files.
The project was created as Mavan project and run using VS Code.
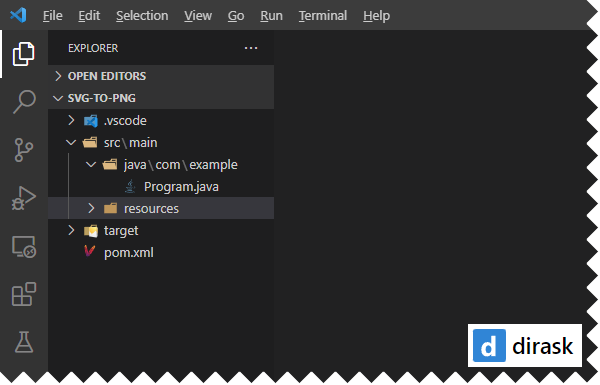
Used SVG file is available here and should be saved under e.g. /path/to/input.svg
(change it in Program.java
file also).
src/main/java/com/example/Program.java
file:
xxxxxxxxxx
1
package com.example;
2
3
import java.io.FileInputStream;
4
import java.io.FileOutputStream;
5
import java.io.IOException;
6
import java.io.OutputStream;
7
8
import org.apache.batik.transcoder.TranscoderException;
9
import org.apache.batik.transcoder.TranscoderInput;
10
import org.apache.batik.transcoder.TranscoderOutput;
11
import org.apache.batik.transcoder.image.PNGTranscoder;
12
13
public class Program {
14
15
public static void main(String[] args) throws IOException, TranscoderException {
16
17
String inputPath = "/path/to/input.svg";
18
String outputPath = "/path/to/output.png";
19
20
float outputWidth = 600.0f;
21
float outputHeight = 600.0f;
22
23
PNGTranscoder transcoder = new PNGTranscoder();
24
transcoder.addTranscodingHint(PNGTranscoder.KEY_WIDTH, outputWidth);
25
transcoder.addTranscodingHint(PNGTranscoder.KEY_HEIGHT, outputHeight);
26
try (FileInputStream inputStream = new FileInputStream(inputPath)) {
27
TranscoderInput input = new TranscoderInput(inputStream);
28
try (OutputStream outputStream = new FileOutputStream(outputPath)) {
29
TranscoderOutput output = new TranscoderOutput(outputStream);
30
transcoder.transcode(input, output);
31
outputStream.flush();
32
}
33
}
34
}
35
}
pom.xml
file:
xxxxxxxxxx
1
2
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
3
4
<modelVersion>4.0.0</modelVersion>
5
6
<groupId>com.example</groupId>
7
<artifactId>svg-to-png</artifactId>
8
9
<name>Example SVG to PNG converter</name>
10
<description>Example SVG to PNG converter using Apache Batik Transcoder.</description>
11
<version>0.0.1-SNAPSHOT</version>
12
<packaging>jar</packaging>
13
14
<properties>
15
<java.version>17</java.version>
16
<maven.compiler.source>${java.version}</maven.compiler.source>
17
<maven.compiler.target>${java.version}</maven.compiler.target>
18
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
19
<project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding>
20
</properties>
21
22
<dependencies>
23
24
<!-- https://mvnrepository.com/artifact/org.apache.xmlgraphics/batik-codec -->
25
<dependency>
26
<groupId>org.apache.xmlgraphics</groupId>
27
<artifactId>batik-codec</artifactId>
28
<version>1.17</version>
29
</dependency>
30
31
<!-- https://mvnrepository.com/artifact/org.apache.xmlgraphics/batik-transcoder -->
32
<dependency>
33
<groupId>org.apache.xmlgraphics</groupId>
34
<artifactId>batik-transcoder</artifactId>
35
<version>1.17</version>
36
</dependency>
37
38
</dependencies>
39
40
<build>
41
<finalName>${project.artifactId}</finalName>
42
<plugins>
43
<plugin>
44
<groupId>org.apache.maven.plugins</groupId>
45
<artifactId>maven-compiler-plugin</artifactId>
46
<version>3.7.0</version>
47
<configuration>
48
<source>${maven.compiler.source}</source>
49
<target>${maven.compiler.target}</target>
50
<verbose>true</verbose>
51
</configuration>
52
</plugin>
53
</plugins>
54
</build>
55
56
</project>
Build project using:
xxxxxxxxxx
1
mvn clean compile
Run project using:
xxxxxxxxxx
1
mvn exec:java -Dexec.mainClass=com.example.Program