EN
Express.js / PostgreSQL - rows pagination
0 points
In this article, we would like to show you how to paginate data rows in PostgreSQL using Express.js.
Note:
Pagination consists in dividing data into pages and sending only those rows that the client currently needs - we do not have to send all data at once.
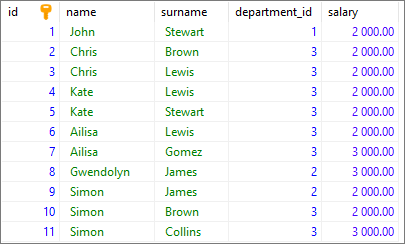
Note: at the end of this article you can find database preparation SQL queries.
xxxxxxxxxx
1
const express = require('express');
2
const { Pool } = require('pg');
3
4
const app = express();
5
6
const pool = new Pool({
7
host: '127.0.0.1',
8
user: 'my_username',
9
database: 'my_database',
10
password: 'my_password',
11
port: 5432,
12
max: 10, // max number of clients in the pool
13
idleTimeoutMillis: 30000, // how long a client is allowed to remain idle before being closed
14
connectionTimeoutMillis: 2000
15
});
16
17
app.get('/users', async (req, res) => {
18
try {
19
const client = await pool.connect(); // creates connection
20
const { page, size } = req.query;
21
const query = `
22
SELECT *
23
FROM "users"
24
ORDER BY "users"."id"
25
LIMIT $2
26
OFFSET (($1 - 1) * $2);
27
`;
28
try {
29
const { rows } = await client.query(query, [page, size]); // sends query
30
res.status(200).json(rows);
31
} finally {
32
await client.release(); // releases connection
33
}
34
} catch (error) {
35
return res.status(500).json(error);
36
}
37
});
38
39
app.listen(3000, () => {
40
console.log('listening at http://localhost:3000');
41
});
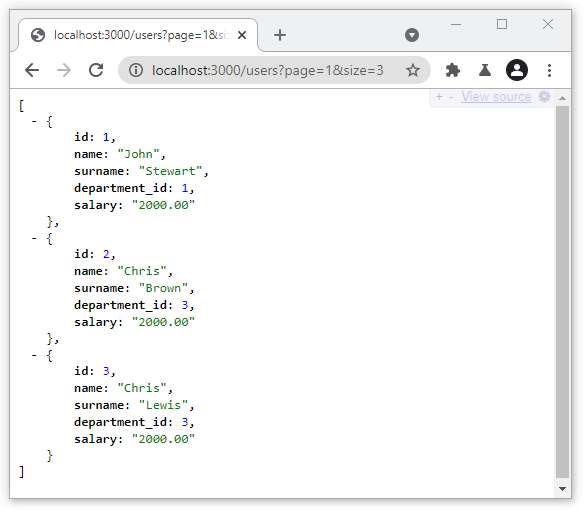
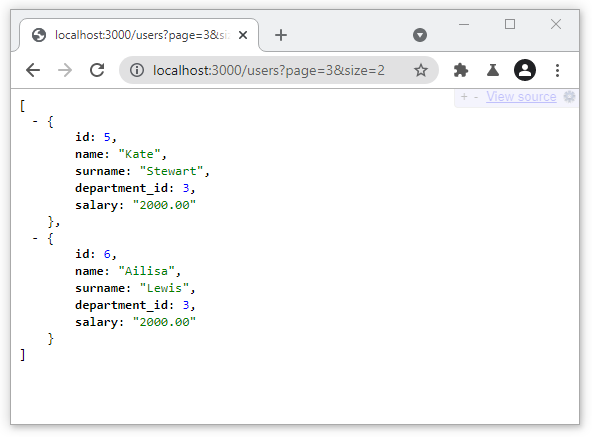
create_tables.sql
file:
xxxxxxxxxx
1
CREATE TABLE "users" (
2
"id" SERIAL,
3
"name" VARCHAR(50) NOT NULL,
4
"surname" VARCHAR(50) NOT NULL,
5
"department_id" INTEGER,
6
"salary" DECIMAL(15,2) NOT NULL,
7
PRIMARY KEY ("id")
8
);
insert_data.sql
file:
xxxxxxxxxx
1
INSERT INTO "users"
2
( "name", "surname", "department_id", "salary")
3
VALUES
4
('John', 'Stewart', 1, '2000.00'),
5
('Chris', 'Brown', 3, '2000.00'),
6
('Chris', 'Lewis', 3, '2000.00'),
7
('Kate', 'Lewis', 3, '2000.00'),
8
('Kate', 'Stewart', 3, '2000.00'),
9
('Ailisa', 'Lewis', 3, '2000.00'),
10
('Ailisa', 'Gomez', 3, '3000.00'),
11
('Gwendolyn', 'James', 2, '3000.00'),
12
('Simon', 'James', 2, '2000.00'),
13
('Simon', 'Brown', 3, '2000.00'),
14
('Simon', 'Collins', 3, '3000.00');