EN
Python - create directory with subdirectory
3 points
In this article, we would like to show you how to create directory with a subdirectory in Python.
Quick solution:
xxxxxxxxxx
1
from pathlib import Path
2
3
Path("C:\\example_directory\example_subdirectory").mkdir(parents=True, exist_ok=True)
Note:
The
exist_ok
parameter was added in Python 3.5. Set it onTrue
so you won't getFileExistsError
if the directory exists.
In this example, we use Path.mkdir()
method from pathlib
module to create a nested directory.
xxxxxxxxxx
1
from pathlib import Path
2
3
Path("C:\\some_path\example_directory\example_subdirectory").mkdir(parents=True, exist_ok=True)
Result:
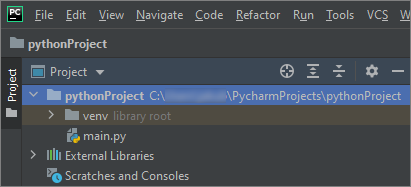
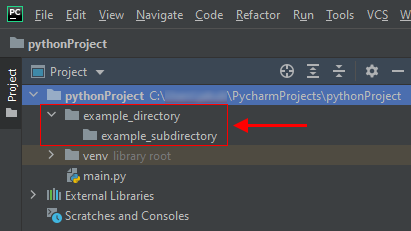
In this example, we use makedirs()
method from os
module to create a nested directory.
xxxxxxxxxx
1
import os
2
3
directory = "C:\\example_path\example_directory\example_subdirectory"
4
5
if not os.path.exists(directory):
6
os.makedirs(directory)