EN
React - how to make animation with CSS transition property
3
points
Hi there! 👋😊
In this article, I would like to show you how to make an animated rotating square in React using transition
property. ⏭
Final effect:
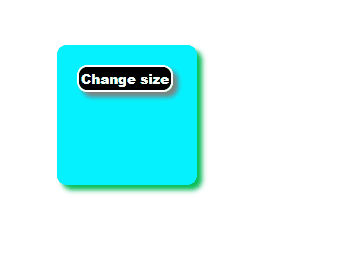
In below example I've created three style objects:
normalStyle
which is the default style of our div element,transformedStyle
which is transformed style of our div element,buttonStyle
which is style of our button element.
The styles of our div have transition
value set to '1s'
. It means our component will change it's property values smoothly, over a given duration (over 1s
). Additional transform
parameter describes moving of an element. In our case transform
rotates the element during 2s
.
Runnable example:
// ONLINE-RUNNER:browser;
// Note: Uncomment import lines during working with JSX Compiler.
// import React from 'react';
// import ReactDOM from 'react-dom';
// --- style objects -------------------------------
const normalStyle = {
margin: '50px',
padding: '20px',
borderRadius: '10px',
width: '100px',
height: '100px',
background: '#06f2ff',
boxShadow: '5px 5px 5px #04bd57',
transition: '1s, transform 2s',
};
const transformedStyle = {
margin: '50px',
padding: '20px',
borderRadius: '10px',
width: '150px',
height: '150px',
background: '#06ff76',
boxShadow: '5px 5px 5px #3085d6',
transition: '1s, transform 2s',
transform: 'rotate(180deg)',
};
const buttonStyle = {
padding: '2px',
outline: 'none',
border: '2px solid white',
background: 'black',
boxShadow: '5px 5px 5px grey',
textShadow: '1px 1px 1px black',
fontWeight: '900',
color: 'white',
borderRadius: '10px',
};
// --- component -------------------------------------
const App = () => {
const [bigSize, setBigSize] = React.useState(false);
const handleClick = () => setBigSize(!bigSize);
return (
<div style={{ height: '300px' }}>
<div style={bigSize ? transformedStyle : normalStyle}>
<button style={buttonStyle} onClick={handleClick}>
Change size
</button>
</div>
</div>
);
};
const root = document.querySelector('#root');
ReactDOM.render(<App />, root );
📝 Note:
If the duration is not specified, thetransition
will have no effect, because the default value is0
.