TypeScript - compile all ts files to one js
In this article, we would like to show how to compile all *.ts
files into one js
file. There are two module
types that let us use single file compilation: amd
and system
. In the below description we used amd
module
.
Quick solution:
1. set in the tsconfig.json
file following compiler options:
{
...
"compilerOptions" : {
...
"module" : "amd",
"outFile" : "./out.js",
...
},
....
}
2. compile TypeScript files running the following command:
tsc
Example project
This section contains example files with compilation and running description.
Node: download example source code from here.
Project directory structure:
/C/
|
+-- Project/
|
+ tsconfig.json
+ Person.ts
+ Printer.ts
+ Main.ts
+ index.htm
1. It is necessary to define proper configuration
tsconfig.json
file:
{
"version" : "3.0.3",
"compilerOptions" : {
"module" : "amd", /* <----------- REQUIRED */
"declaration" : true,
"outFile" : "./out.js", /* <----------- REQUIRED */
"baseUrl" : "./",
"sourceMap" : true, /* <----------- only to debug ts files */
"alwaysStrict" : true,
"removeComments" : true
},
"include": [
"./**/*.ts"
],
"exclude": [
"./out.d.ts"
]
}
Note:
"outFile" : "./out.js"
defines compilation result (one file).
2. Now we can prepare some *.ts
files that will be compiled into one file.
Person.ts
file:
export class Person {
public constructor(public name: string, public age: number) {
// nothing here ...
}
public toString(): string {
return `{ Name: ${this->name}, Age: ${this->age} }`;
}
}
Printer.ts
file:
import { Person } from "./Person";
export class Printer {
public static printPerson(person: Person): void {
console.log(person.toString());
}
public static printPersons(persons: Array<Person>): void {
for(let entry of persons) {
this.printPerson(entry);
}
}
}
Main.ts
file:
import { Person } from "./Person";
import { Printer } from "./Printer";
const persons = new Array<Person>();
persons.push(new Person('John', 30));
persons.push(new Person('Adam', 23));
persons.push(new Person('Kate', 41));
Printer.printPersons(persons);
3. Each web page requires index.html
that runs a page - in this case, is the same.
require.js
was attached as first because out.js
file depends on it. out.js
contains all ts
source code compiled into a single js
file. Last important thing is to tell the application to run the web page using the main module - in our case, the main module is Main
, so require(['Main'])
was called.
index.htm
file:
<!doctype html>
<html>
<head>
<script src="https://requirejs.org/docs/release/2.3.6/comments/require.js"></script>
<!-- or: https://requirejs.org/docs/release/2.3.6/minified/require.js -->
<script src="out.js"></script>
</head>
<body>
<script>
require(['Main']);
</script>
</body>
</html>
Note: above source code uses https://requirejs.org/ modules loader.
4. Compilation and running
Simple steps:
- open command line (e.g. Windows Command Prompt or Bash),
- go to the project directory,
- run
tsc
command to compile all files to one, - open
index.htm
in web browser
Console preview:
$ cd /C/Project
$ tsc
Directory screenshot:
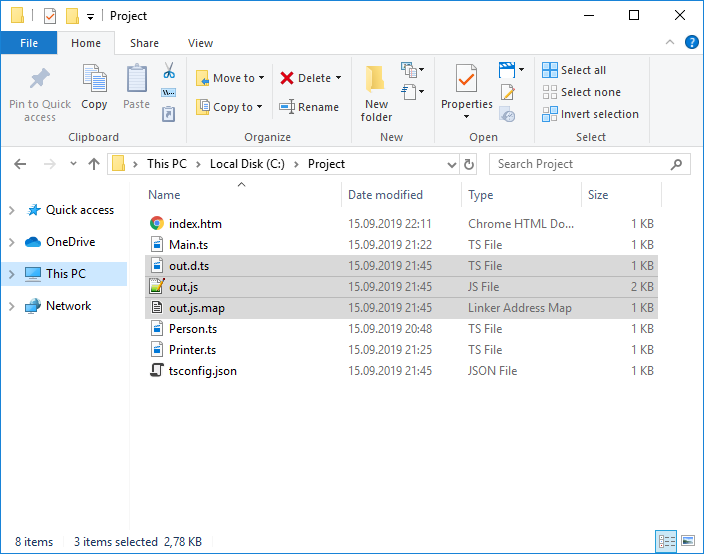
Notes:
- after
tsc
command run we should see 3 new files:
out.d.ts
contains all declarations,
(useful for intellisense and intengrationout.js
file with other typescript projects)out.js.map
contains development mapping for debug process,out.js
contains all sourcecode transpiled to single javascript file.
Web Browser preview:
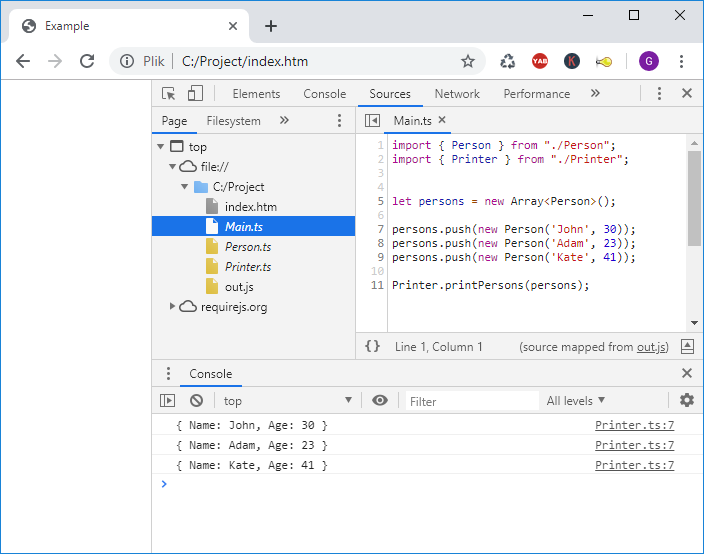
Note: even website uses one js file now, DevTools shows multiple ts files to make reading source code easier for programmer - map file provides this feature
5. Project source code
Download all source code from one place here.