EN
Node.js - how to use .env
0 points
In this article, we would like to show you how to use .env in Node.js.
The .env file is used to store environment variables. Environment variables are used to protect the sensitive data (like API keys) that shouldn't be visible directly in the code.
To use environment variables, install dotenv package with the following command in the console:
xxxxxxxxxx
1
npm install dotenv
Import dotenv
in your project and use dotenv.config()
to make it work.
xxxxxxxxxx
1
const dotenv = require('dotenv');
2
dotenv.config();
The next step is to create .env file. Below you can see the example project structure.
xxxxxxxxxx
1
/app/
2
├── node_modules/
3
├── .env
4
├── index.js
5
├── package-lock.json
6
└── package.json
There are two ways of setting the environment variables.
1. Directly in .env file:
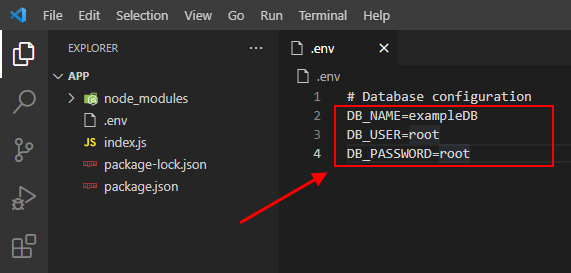
Note:
The
#
in .env file is used for comments.
2. From the code:
xxxxxxxxxx
1
const dotenv = require('dotenv');
2
dotenv.config();
3
4
// Set DB_NAME environment variable
5
process.env['DB_NAME'] = 'myDatabase';
To access keys and values you defined in your .env
file use the process.env
.
Practical example:
xxxxxxxxxx
1
const dotenv = require('dotenv');
2
dotenv.config();
3
4
// get database name from .env file
5
console.log(process.env.DB_NAME);
Output:
xxxxxxxxxx
1
exampleDB