EN
MySQL - Update multiple rows at once
0 points
In this article, we would like to show you how to update multiple rows at once in MySQL.
Quick solution:
xxxxxxxxxx
1
UPDATE `table_name`
2
SET `column1` = value1, `column2` = value2, `columnN` = valueN
3
WHERE condition;
To show you how to update multiple rows at once, we will use the following users
table:
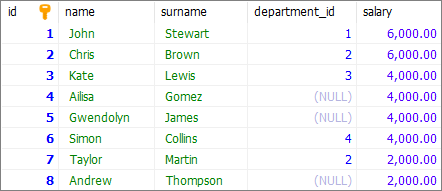
Note:
At the end of this article you can find database preparation SQL queries.
In this example, we will update department_id
and salary
value of the first three users from the users
table.
Query
xxxxxxxxxx
1
UPDATE `users`
2
SET `department_id` = 5, `salary` = 6000
3
WHERE `id` <= 3;
or
xxxxxxxxxx
1
UPDATE `users`SET `department_id` = 5, `salary` = 6000 WHERE `id` = 1;
2
UPDATE `users`SET `department_id` = 5, `salary` = 6000 WHERE `id` = 2;
3
UPDATE `users`SET `department_id` = 5, `salary` = 6000 WHERE `id` = 3;
Result:
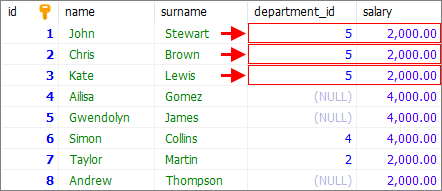
create_tables.sql
file:
xxxxxxxxxx
1
CREATE TABLE `users` (
2
`id` INT(10) UNSIGNED NOT NULL AUTO_INCREMENT,
3
`name` VARCHAR(50) NOT NULL,
4
`surname` VARCHAR(50) NOT NULL,
5
`department_id` INT(10) UNSIGNED,
6
`salary` DECIMAL(15,2) NOT NULL,
7
PRIMARY KEY (`id`)
8
);
insert_data.sql
file:
xxxxxxxxxx
1
INSERT INTO `users`
2
( `name`, `surname`, `department_id`, `salary`)
3
VALUES
4
('John', 'Stewart', 1, '6000'),
5
('Chris', 'Brown', 2, '6000'),
6
('Kate', 'Lewis', 3, '4000'),
7
('Ailisa', 'Gomez', NULL, '4000'),
8
('Gwendolyn', 'James', NULL, '4000'),
9
('Simon', 'Collins', 4, '4000'),
10
('Taylor', 'Martin', 2, '2000'),
11
('Andrew', 'Thompson', NULL, '2000');