EN
MySQL - Select from multiple tables (using JOIN)
0
points
In this article, we would like to show you how to select from multiple tables using JOIN
clause in MySQL.
Quick solution:
SELECT `table1`.`column1`,`table2`.`column2`
FROM `table1`
JOIN `table2` ON `table1`.`column_name` = `table2`.`reference_column_name`;
Practical example
To show how to count rows per day, we will use the following tables:
groups table:
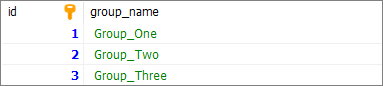
users table:
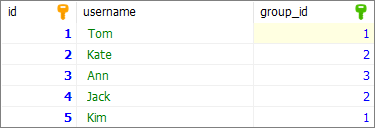
Note:
At the end of this article you can find database preparation SQL queries.
Example
In this example, we will display the following columns from users
table:
id
,username
,group_id
,
and the following column from groups
table:
group_name
We will use aliases:
u
- forusers
table,g
- forgroups
table.
In our example group_id
is a reference column for `groups`.`id`
(g.`id`
).
Query:
SELECT u.`id`, u.`username`, u.`group_id`, g.`group_name`
FROM `users` AS u
JOIN `groups` AS g ON u.`group_id` = g.`id`;
Result:
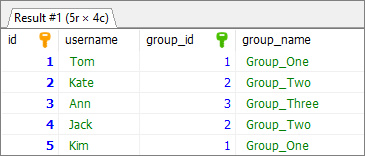
Database preparation
create_tables.sql
file:
CREATE TABLE `groups` (
`id` INT(10) UNSIGNED NOT NULL AUTO_INCREMENT,
`group_name` VARCHAR(50) NOT NULL,
PRIMARY KEY (`id`)
);
CREATE TABLE `users` (
`id` INT(10) UNSIGNED NOT NULL AUTO_INCREMENT,
`username` VARCHAR(50) NOT NULL,
`group_id` INT(10) UNSIGNED,
PRIMARY KEY (`id`),
FOREIGN KEY (`group_id`) REFERENCES `groups`(`id`)
);
insert_data.sql
file:
INSERT INTO `groups`
(`group_name`)
VALUES
('Group_One'),
('Group_Two'),
('Group_Three');
INSERT INTO `users`
(`username`, `group_id`)
VALUES
('Tom', 1),
('Kate', 2),
('Ann', 3),
('Jack', 2),
('Kim', 1);