EN
JavaScript - track last 10 site loading times and print statistics
4
points
This article contains logic that lets to print page loading times.
As page loading times, we understand the time necessary to load only site entry point (it means: index.html
or index.php
or ... - depending on technology).
Results presented are in the following way:
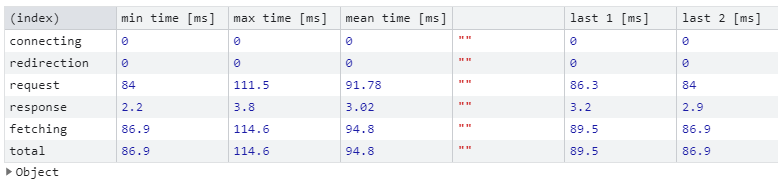
Usage:
- paste below script to
body
element, - open Console in the Google Chrome DevTools,
- refresh the page many times to collect loading times (refresh more than 10 times).
Hint: change
var historySize = 10;
to collect the different amounts of the last times.
Source code ():
// storage logic
function readArray(name) {
var json = localStorage.getItem(name);
if (json) {
return JSON.parse(json);
}
return null;
}
function saveArray(name, array) {
var json = JSON.stringify(array);
localStorage.setItem(name, json);
}
// performance logic
function checkPerformance() {
if (performance.getEntries) {
var entries = performance.getEntries();
if (entries.length > 0) {
return entries[0];
}
}
return null;
}
function getTimes() {
var performance = checkPerformance();
if (performance) {
return {
connectingDuration: performance.connectEnd - performance.connectStart,
redirectionDuration: performance.redirectEnd - performance.redirectStart,
requestDuration: performance.responseStart - performance.requestStart,
responseDuration: performance.responseEnd - performance.responseStart,
fetchingDuration: performance.responseEnd - performance.requestStart, // request time + TTFB + response time (downloading)
totalDuration: performance.responseEnd - (performance.redirectStart || performance.connectStart)
};
}
return null;
}
// arrays logic
function createArray(count) {
var array = Array(count);
for (var i = 0; i < count; ++i) {
array[i] = 0;
}
return array;
}
function resizeArray(array, size) {
var changeSize = size - array.length;
if (changeSize > 0) {
return array.concat(createArray(changeSize));
} else {
return array.slice(0, size);
}
}
// math logic
function isLesser(result, value) {
return result < value;
}
function isBigger(result, value) {
return result > value;
}
function roundNumber(value, precision) {
var power = Math.pow(10, precision);
return Math.round(value * power) / power;
}
function calculateSum(array) {
var sum = 0;
for (var i = 0; i < array.length; ++i) {
sum += array[i];
}
return sum;
}
function calculateMean(array) {
var sum = calculateSum(array);
return sum / array.length;
}
function findValue(array, compare) {
if (array.length > 0) {
var result = array[0];
for (var i = 1; i < array.length; ++i) {
var value = array[i];
if (compare(value, result)) {
result = value;
}
}
return result;
}
return NaN;
}
// processing logic
function updateValues(historySize, parameterName, parameterValue) {
var storedValues = readArray(parameterName + 's');
if (storedValues == null) {
storedValues = createArray(historySize);
} else {
if (storedValues.length != historySize) {
storedValues = resizeArray(storedValues, historySize);
}
}
storedValues.shift();
storedValues.push(parameterValue);
saveArray(parameterName + 's', storedValues);
return storedValues;
}
// Usage example:
var historySize = 10;
window.addEventListener('load', function() {
var currentTimes = getTimes();
if (currentTimes) {
var gatheredTimes = {};
for (var parameterName in currentTimes) {
var storedValues = updateValues(historySize, parameterName, currentTimes[parameterName])
var minTime = findValue(storedValues, isLesser);
var maxTime = findValue(storedValues, isBigger);
var meanTime = calculateMean(storedValues);
var parameterTimes = {
'min time [ms]': roundNumber(minTime, 3),
'max time [ms]': roundNumber(maxTime, 3),
'mean time [ms]': roundNumber(meanTime, 3),
'': '' // used as separator
};
for (var i = 0; i < historySize; ++i) {
parameterTimes['last ' + (i + 1) + ' [ms]'] = roundNumber(storedValues[historySize - i - 1], 3);
}
gatheredTimes[parameterName.substring(0, parameterName.length - 8)] = parameterTimes;
}
console.table(gatheredTimes);
}
});
See also