EN
JavaScript - rotate localized vector 2D to left direction (counterclockwise direction)
7
points
In this short article, we would like to show how to rotate any localized vector 2D to the left side (90 degrees) using Javascript.
Quick solution:
function rotateLeft(vector) {
var a = vector.a;
var b = vector.b;
return {
a: { x: -a.y, y: a.x }, // check: https://dirask.com/posts/1GoW61
b: { x: -b.y, y: b.x }
};
}
var vector1 = {a: {x: 1, y: 2}, b: {x: 2, y: 4}}; // vector [A1, B1] = ( 1, 2) -> ( 2, 4)
var vector2 = rotateLeft(vector1); // vector [A2, B2] = (-2, 1) -> (-4, 2)
Rotation preview:
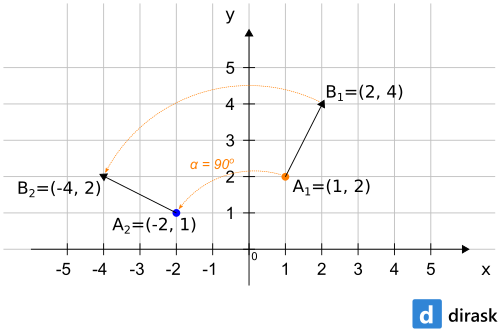
Practical example
// ONLINE-RUNNER:browser;
function rotateLeft(vector) {
var a = vector.a;
var b = vector.b;
return {
a: { x: -a.y, y: a.x }, // check: https://dirask.com/posts/1GoW61
b: { x: -b.y, y: b.x }
};
}
// Helper methods:
function printPoint(point) {
return '(' + point.x + ', ' + point.y + ')';
}
function printVector(name, vector) {
console.log(name + ': ' + printPoint(vector.a) + ' -> ' + printPoint(vector.b));
}
// Usage example:
// direction:
var vector1 = { // ↑
a: {x: 0, y: 1}, // a = beginning point
b: {x: 0, y: 2} // b = ending point (arrow/head)
};
var vector2 = rotateLeft(vector1); // ←
var vector3 = rotateLeft(vector2); // ↓
var vector4 = rotateLeft(vector3); // →
printVector('vector1', vector1); // ( 0, 1) -> ( 0, 2) ↑
printVector('vector2', vector2); // (-1, 0) -> (-2, 0) ←
printVector('vector3', vector3); // ( 0, -1) -> ( 0, -2) ↓
printVector('vector4', vector4); // ( 1, 0) -> ( 2, 0) →