EN
JavaScript - draw image on canvas element
3 points
Quick solution:
xxxxxxxxxx
1
// drawing image at position 50x50 px with size 140x140 px
2
context.drawImage(image, 50, 50, 140, 140);
Using JavaScript canvas element context it is possible to draw image in following way.
With this approach HTMLImageElement
type handle is necessary.
Avaialble approaches to get element handle are:
- with
document.querySelector()
,docuemnt.getElementById()
or other similar method, - with
document.createElement('img')
execution, - with
new Image()
execution.
Following example shows how to use new Image()
approach:
xxxxxxxxxx
1
2
<html>
3
<head>
4
<style>
5
6
#my-canvas { border: 1px solid gray; }
7
8
</style>
9
</head>
10
<body>
11
<canvas id="my-canvas" width="200" height="200"></canvas>
12
<script>
13
14
var canvas = document.querySelector('#my-canvas');
15
var context = canvas.getContext('2d');
16
17
var image = new Image();
18
19
image.onload = function() {
20
context.drawImage(image, 50, 50, 140, 140);
21
};
22
23
image.onerror = function() {
24
context.fillStyle = 'red';
25
context.font = '16px Arial';
26
context.fillText('Image loading error!', 10, 30);
27
};
28
29
image.src = 'https://dirask.com/static/bucket/1574890428058-BZOQxN2D3p--image.png';
30
31
</script>
32
</body>
33
</html>
Used resources:
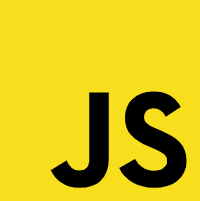