EN
CSS - responsive styles for desktop and mobile (mobile first approach)
3 points
In this short article, we want to show how to create responsive desktop and mobile styles for a simple page layout using @media
queries in CSS.
The below example uses a mobile-first approach - it means at first we have created styles for mobile and later some of them were overwritten using @media
block to achieve desktop styles.
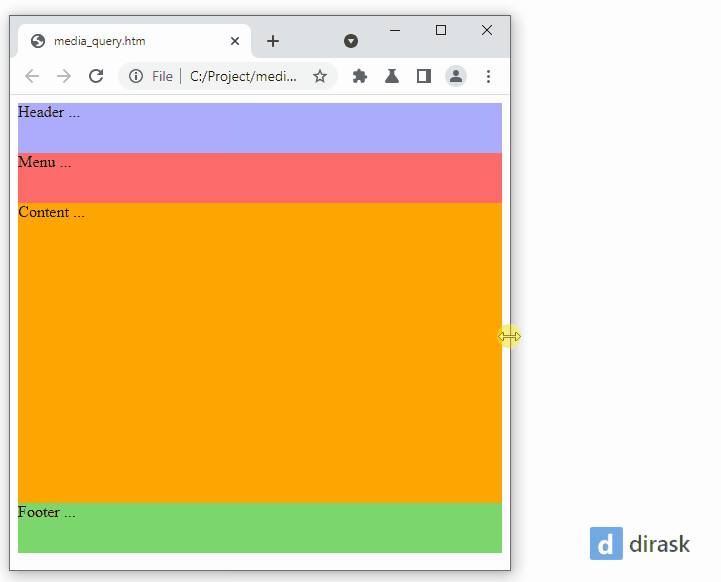
Note: run the below example and change the web browser window size to bigger - you will see the effect.
Practical example:
xxxxxxxxxx
1
2
<html>
3
<head>
4
<style>
5
6
/* Mobile first approach */
7
8
/* Default styles for Mobile */
9
10
div.header {
11
background: #adadff;
12
height: 50px;
13
}
14
15
div.container {
16
height: auto;
17
display: block;
18
}
19
20
div.menu {
21
background: #ff6b6b;
22
flex: none;
23
height: 50px;
24
}
25
26
div.content {
27
background: orange;
28
flex: none;
29
height: 300px;
30
}
31
32
div.footer {
33
background: #7dd96d;
34
height: 50px;
35
}
36
37
/* Desktop styles for screen width > 550px */
38
39
@media (min-width: 550px) {
40
41
/* below styles overwrite mobile styles */
42
43
div.container {
44
display: flex;
45
}
46
47
div.menu {
48
flex: 0 0 200px;
49
height: auto;
50
}
51
52
div.content {
53
flex: auto;
54
}
55
}
56
57
</style>
58
</head>
59
<body>
60
<div class="header">Header ...</div>
61
<div class="container">
62
<div class="menu">Menu ...</div>
63
<div class="content">Content ...</div>
64
</div>
65
<div class="footer">Footer ...</div>
66
</body>
67
</html>
68