EN
JavaScript - draw Rastrigin 2D on canvas element
13 points
In this short article, we would like to show a simple way how to plot a Rastrigin 2D chart on HTML5 canvas element using JavaScript.
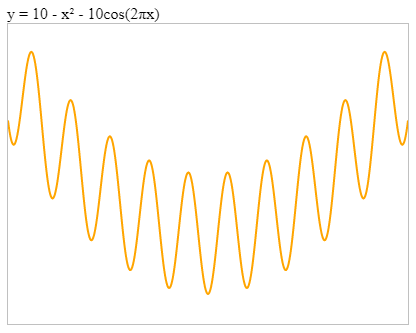
Used formula:
xxxxxxxxxx
1
const rastrigin2D = function(x) {
2
return 10 + x * x - 10 * Math.cos(2 * Math.PI * x);
3
};
4
5
6
// Usage example:
7
8
console.log(rastrigin2D(-5.12)); // 28.924713725785892
9
console.log(rastrigin2D(-2.56)); // 25.851364858882512
10
console.log(rastrigin2D(0.0)); // 0
11
console.log(rastrigin2D(+2.56)); // 25.851364858882512
12
console.log(rastrigin2D(+5.12)); // 28.924713725785892
xxxxxxxxxx
1
2
<html>
3
<head>
4
<style> canvas { border: 1px solid silver; } </style>
5
</head>
6
<body>
7
<div>y = 10 - x² - 10cos(2πx)</div>
8
<canvas id="canvas" width="400" height="300"></canvas>
9
<script>
10
11
function Calculator(canvas, x1, x2, y1, y2, func) {
12
var cWidth = canvas.width;
13
var cHeight = canvas.height;
14
var xRange = x2 - x1;
15
var yRange = y2 - y1;
16
this.calculatePoint = function(x) {
17
var y = func(x);
18
// chart will be reversed horizontaly because of reversed canvas pixels
19
var nx = (x - x1) / xRange; // normalized x
20
var ny = 1.0 - (y - y1) / yRange; // normalized y
21
return {
22
x: nx * cWidth,
23
y: ny * cHeight
24
};
25
};
26
}
27
28
function Chart(canvas, x1, x2, y1, y2, dx, func) {
29
var context = canvas.getContext('2d');
30
var calculator = new Calculator(canvas, x1, x2, y1, y2, func);
31
this.drawChart = function(color, width) {
32
var a = calculator.calculatePoint(x1);
33
var b = calculator.calculatePoint(x2);
34
context.beginPath();
35
context.moveTo(a.x, a.y);
36
for (var x = x1 + dx; x < x2; x += dx) {
37
var point = calculator.calculatePoint(x);
38
context.lineTo(point.x, point.y);
39
}
40
context.lineTo(b.x, b.y);
41
context.strokeStyle = color || 'black';
42
context.lineWidth = width || 1;
43
context.stroke();
44
};
45
}
46
47
48
// Usage example:
49
50
var canvas = document.querySelector('#canvas');
51
52
var rastrigin2D = function(x) {
53
return 10 + x * x - 10 * Math.cos(2 * Math.PI * x);
54
};
55
56
// min max min max (x step)
57
// x x y y dx
58
var chart = new Chart(canvas, -5.12, +5.12, -5.0, +45.0, +0.02, rastrigin2D);
59
60
chart.drawChart('orange', 2);
61
62
</script>
63
</body>
64
</html>