EN
JavaScript - read image from clipboard as Data URLs encoded with Base64
4 points
In this short article we will see how in JavaScript read image from clipboard as Data URLs encoded with Base64.
Very important thing is: this feature is available in the newest browsers but it can be treated as additional feature that helps user working ergonomics.
How to use it?:
- copy some bitmap to clipboard
(it is good to open some image editor and copy selected image area, use some snipping tool or just press Print Screen key), - run below example,
- press
Paste image bitmap from clipboard
button.
Practical example:
xxxxxxxxxx
1
2
<html>
3
<body>
4
<div>
5
<img id="image" style="border: 1px solid silver; width: 320px; height: 240px" />
6
<br /><br />
7
<button onclick="pasteImageBitmap()">Paste image bitmap from clipboard</button>
8
</div>
9
<script>
10
11
var ClipboardUtils = new function() {
12
var permissions = {
13
'image/bmp': true,
14
'image/gif': true,
15
'image/png': true,
16
'image/jpeg': true,
17
'image/tiff': true
18
};
19
function getType(types) {
20
for (var j = 0; j < types.length; ++j) {
21
var type = types[j];
22
if (permissions[type]) {
23
return type;
24
}
25
}
26
return null;
27
}
28
function getItem(items) {
29
for (var i = 0; i < items.length; ++i) {
30
var item = items[i];
31
if(item) {
32
var type = getType(item.types);
33
if(type) {
34
return item.getType(type);
35
}
36
}
37
}
38
return null;
39
}
40
function readFile(file, callback) {
41
if (window.FileReader) {
42
var reader = new FileReader();
43
reader.onload = function() {
44
callback(reader.result, null);
45
};
46
reader.onerror = function() {
47
callback(null, 'Incorrect file.');
48
};
49
reader.readAsDataURL(file);
50
} else {
51
callback(null, 'File API is not supported.');
52
}
53
}
54
this.readImage = function(callback) {
55
if (navigator.clipboard) {
56
var promise = navigator.clipboard.read();
57
promise
58
.then(function(items) {
59
var promise = getItem(items);
60
if (promise == null) {
61
callback(null, null);
62
return;
63
}
64
promise
65
.then(function(result) {
66
readFile(result, callback);
67
})
68
.catch(function(error) {
69
callback(null, error || 'Clipboard reading error.');
70
});
71
})
72
.catch(function(error) {
73
callback(null, error || 'Clipboard reading error.');
74
});
75
} else {
76
callback(null, 'Clipboard API is not supported.');
77
}
78
};
79
};
80
81
// Usage example:
82
83
var image = document.querySelector('#image');
84
85
function pasteImageBitmap() {
86
ClipboardUtils.readImage(function(data, error) {
87
if (error) {
88
console.error(error);
89
return;
90
}
91
if (data) {
92
image.src = data;
93
return;
94
}
95
console.log('Image bitmap is not avaialble - copy it to clipboard.');
96
});
97
}
98
99
</script>
100
</body>
101
</html>
Copy this graphic to system clipboard.
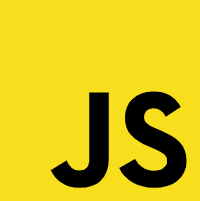