EN
Node.js - PostgreSQL - count distinct values
0
points
In this article, we would like to show you how to count distinct values in the PostgreSQL database using Node.js.
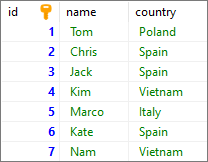
Note: at the end of this article you can find database preparation SQL queries.
const { Client } = require('pg');
const client = new Client({
host: '127.0.0.1',
user: 'postgres',
database: 'database_name',
password: 'password',
port: 5432,
});
const countUniqueCountries = async () => {
const query = `SELECT COUNT(DISTINCT("country")) AS "unique_countries"
FROM "users";`;
try {
await client.connect(); // creates connection
const { rows } = await client.query(query); // sends query
console.log(rows);
} catch (error) {
console.error(error.stack);
} finally {
await client.end(); // closes connection
}
};
countUniqueCountries();
Result:
[ { unique_countries: '4' } ]
Database preparation
create_tables.sql
file:
CREATE TABLE "users" (
"id" SERIAL,
"name" VARCHAR(100) NOT NULL,
"country" VARCHAR(15) NOT NULL,
PRIMARY KEY ("id")
);
insert_data.sql
file:
INSERT INTO "users"
("name", "country")
VALUES
('Tom', 'Poland'),
('Chris', 'Spain'),
('Jack', 'Spain'),
('Kim', 'Vietnam'),
('Marco', 'Italy'),
('Kate', 'Spain'),
('Nam', 'Vietnam');