EN
Node.js - PostgreSQL - find row with null value in column
0 points
In this article, we would like to show you how to find the row with a null value in a column in the PostgreSQL database using Node.js.
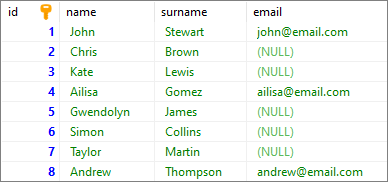
Note: at the end of this article you can find database preparation SQL queries.
xxxxxxxxxx
1
const { Client } = require('pg');
2
3
const client = new Client({
4
host: '127.0.0.1',
5
user: 'postgres',
6
database: 'database_name',
7
password: 'password',
8
port: 5432,
9
});
10
11
const getRowWithoutEmail = async () => {
12
const query = `SELECT *
13
FROM "users"
14
WHERE "email" IS NULL;`;
15
try {
16
await client.connect(); // creates connection
17
const { rows } = await client.query(query); // sends query
18
console.table(rows);
19
} catch (error) {
20
console.error(error.stack);
21
} finally {
22
await client.end(); // closes connection
23
}
24
};
25
26
getRowWithoutEmail();
Result:
xxxxxxxxxx
1
┌─────────┬────┬─────────────┬───────────┬───────┐
2
│ (index) │ id │ name │ surname │ email │
3
├─────────┼────┼─────────────┼───────────┼───────┤
4
│ 0 │ 2 │ 'Chris' │ 'Brown' │ null │
5
│ 1 │ 3 │ 'Kate' │ 'Lewis' │ null │
6
│ 2 │ 5 │ 'Gwendolyn' │ 'James' │ null │
7
│ 3 │ 6 │ 'Simon' │ 'Collins' │ null │
8
│ 4 │ 7 │ 'Taylor' │ 'Martin' │ null │
9
└─────────┴────┴─────────────┴───────────┴───────┘
xxxxxxxxxx
1
CREATE TABLE "users" (
2
"id" SERIAL,
3
"name" VARCHAR(50) NOT NULL,
4
"surname" VARCHAR(50) NOT NULL,
5
"email" VARCHAR(50),
6
PRIMARY KEY ("id")
7
);
insert_data.sql
file:
xxxxxxxxxx
1
INSERT INTO "users"
2
( "name", "surname", "email")
3
VALUES
4
('John', 'Stewart', 'john@email.com'),
5
('Chris', 'Brown', NULL),
6
('Kate', 'Lewis', NULL),
7
('Ailisa', 'Gomez', 'ailisa@email.com'),
8
('Gwendolyn', 'James', NULL),
9
('Simon', 'Collins', NULL),
10
('Taylor', 'Martin', NULL),
11
('Andrew', 'Thompson', 'andrew@email.com');
Native SQL query (used in the above example):
xxxxxxxxxx
1
SELECT *
2
FROM "users"
3
WHERE "email" IS NULL