TypeScript - create common project used by multiple projects (works only in web browser)
In this article, we would like to show how to create common project that is used by multiple projects using TypeScript.
In the article, presented solution do not compile common project as separated *.js bundle. It compiles all used *.js files into each project output *.js bundles.
Hint: to know how to use compiled files in the web browser check this article.
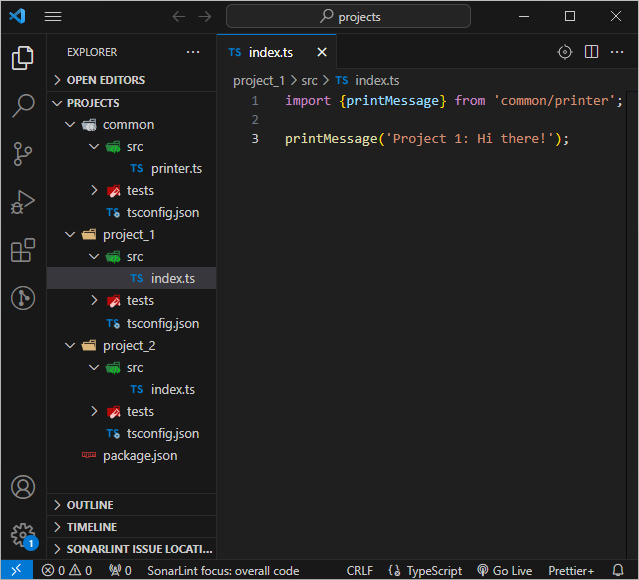
Note: it is good to add Path Autocomplete plugin to get better intellisende with common code inside projects when we use VS Code.
package.json
file:
xxxxxxxxxx
{
"name": "module_1",
"version": "1.0.0",
"description": "",
"scripts": {
"common:compile": "tsc -p common/tsconfig.json",
"project_1:compile": "tsc -p project_1/tsconfig.json",
"project_2:compile": "tsc -p project_2/tsconfig.json"
},
"author": "",
"license": "ISC"
}
src/printer.ts
file:
xxxxxxxxxx
export const printMessage = (message: string): void => {
console.log(message);
};
tsconfig.json
file:
xxxxxxxxxx
{
"compilerOptions": {
"strict": true,
"target": "ES6",
"module": "AMD",
"moduleResolution": "node",
"removeComments": false,
"sourceMap": true,
"baseUrl": "./src",
"outFile": "out.js"
},
"include": [
"./src/**/*",
"./tests/**/*"
]
}
Hint: this configuration is used only to force source code checking by IDE in
common
project insrc/
andtests/
directories.
src/index.ts
file:
xxxxxxxxxx
import {printMessage} from 'common/printer';
printMessage('Project 1: Hi there!');
Hint: to compile source code use
npm run project_1:compile
command.
tsconfig.json
file:
xxxxxxxxxx
{
"compilerOptions": {
"strict": true,
"target": "ES6",
"module": "AMD",
"moduleResolution": "node",
"removeComments": false,
"sourceMap": true,
"baseUrl": "./src",
"outFile": "out.js",
"paths": {
"common/*": ["../../common/src/*"]
}
},
"include": [
"../../common/src/**/*",
"./src/**/*",
"./tests/**/*"
]
}
src/index.ts
file:
xxxxxxxxxx
import {printMessage} from 'common/printer';
printMessage('Project 2: Hi there!');
Hint: to compile source code use
npm run project_2:compile
command.
tsconfig.json
file:
xxxxxxxxxx
{
"compilerOptions": {
"strict": true,
"target": "ES6",
"module": "AMD",
"moduleResolution": "node",
"removeComments": false,
"sourceMap": true,
"baseUrl": "./src",
"outFile": "out.js",
"paths": {
"common/*": ["../../common/src/*"]
}
},
"include": [
"../../common/src/**/*",
"./src/**/*",
"./tests/**/*"
]
}