Create simple login form in React
Hello there! 👋😊
In this article, I would like to show you how to make a simple login form in React.
Final effect:
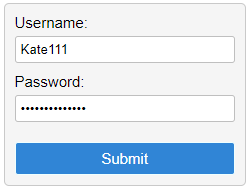
Below I will try to explain to you how to create such a form in a few steps.
The whole structure of the example consists of the Field
component, which will serve as a reusable code ♻, and the Form
, which puts it all together.
In our case, the style
property was used for styling. 🎨
To get the values entered by the user in the inputs, I've used the useRef
hook, with which we can easily obtain a handle to a DOM element and retrieve the current value.
Using these values, we build an object that we can then send to the server after handling onSubmit
. 📤
To prevent the page reloading on onSubmit
event, we explicitly called preventDefault()
method.
📝Note:
By default,
ref
property is reserved by React, so we should use theforwardRef
function.
Runnable example:
// ONLINE-RUNNER:browser;
// Note: Uncomment import lines during working with JSX Compiler.
// import React from 'react';
// import ReactDOM from 'react-dom';
const appStyle = {
height: '250px',
display: 'flex'
};
const formStyle = {
margin: 'auto',
padding: '10px',
border: '1px solid #c9c9c9',
borderRadius: '5px',
background: '#f5f5f5',
width: '220px',
display: 'block'
};
const labelStyle = {
margin: '10px 0 5px 0',
fontFamily: 'Arial, Helvetica, sans-serif',
fontSize: '15px',
};
const inputStyle = {
margin: '5px 0 10px 0',
padding: '5px',
border: '1px solid #bfbfbf',
borderRadius: '3px',
boxSizing: 'border-box',
width: '100%'
};
const submitStyle = {
margin: '10px 0 0 0',
padding: '7px 10px',
border: '1px solid #efffff',
borderRadius: '3px',
background: '#3085d6',
width: '100%',
fontSize: '15px',
color: 'white',
display: 'block'
};
const Field = React.forwardRef(({label, type}, ref) => {
return (
<div>
<label style={labelStyle} >{label}</label>
<input ref={ref} type={type} style={inputStyle} />
</div>
);
});
const Form = ({onSubmit}) => {
const usernameRef = React.useRef();
const passwordRef = React.useRef();
const handleSubmit = e => {
e.preventDefault();
const data = {
username: usernameRef.current.value,
password: passwordRef.current.value
};
onSubmit(data);
};
return (
<form style={formStyle} onSubmit={handleSubmit} >
<Field ref={usernameRef} label="Username:" type="text" />
<Field ref={passwordRef} label="Password:" type="password" />
<div>
<button style={submitStyle} type="submit">Submit</button>
</div>
</form>
);
};
// Usage example:
const App = () => {
const handleSubmit = data => {
const json = JSON.stringify(data, null, 4);
console.clear();
console.log(json);
};
return (
<div style={appStyle}>
<Form onSubmit={handleSubmit} />
</div>
);
};
const root = document.querySelector('#root');
ReactDOM.render(<App />, root );
So that's my version of the simple login form in React.
If you have any questions feel free to ask in our questions section, we'll be happy to help you. 😊
Happy coding!