PL
React - własny animowany wykres słupkowy
3
points
W tym krótkim artykule chcielibyśmy pokazać, jak stworzyć animowany wykres słupkowy w Reakcie przy użyciu elementów div.
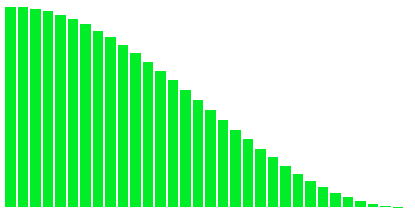
Szybkie rozwiązanie:
// ONLINE-RUNNER:browser;
// import React from 'react';
// import ReactDOM from 'react-dom';
// ---------------------------------
const containerStyle = {
padding: '0 1px',
background: '#ffffff',
flex: '1'
};
const spaceStyle = {
background: '#ffffff',
transition: '0.3s'
};
const barStyle = {
background: '#00cc00',
transition: '0.3s'
};
const Bar = ({value}) => {
return (
<div style={containerStyle}>
<div style={{...spaceStyle, height: `${100 - value}%`}} />
<div style={{...barStyle, height: `${value}%`}} />
</div>
);
};
const chartStyle = {
width: '400px',
height: '300px',
display: 'flex',
overflow: 'hidden'
};
const Chart = ({data}) => {
return (
<div style={chartStyle}>
{data.map((value, index) => <Bar key={index} value={value} />)}
</div>
);
};
// ---------------------------------
const calculateData = (xOffset) => {
const data = [ ];
for (var x = 0; x < 3.1415; x += 0.1) {
const y = Math.cos(x + xOffset) + 1;
data.push(50 * y);
}
return data;
};
const App = () => {
const [data, setData] = React.useState(() => calculateData(0));
const xOffsets = [0, 0.7853, 1.5707, 2.3559, 3.1415];
return (
<div>
<Chart data={data} />
<br />
<div>
<span>xOffset: </span>
{xOffsets.map(xOffset => {
const handleClick = () => setData(calculateData(xOffset));
return (
<button key={xOffset} onClick={handleClick}>{xOffset}</button>
);
})}
</div>
</div>
);
};
const root = document.querySelector('#root');
ReactDOM.render(<App />, root);