EN
C# / .NET - Math.Log() method example
0
points
The Math.Log()
method returns the natural logarithm (base e) of a number.
using System;
public class Program
{
public static void Main(string[] args)
{
// Natural logarithm (logarithm with base e):
// x y
Console.WriteLine( Math.Log( 1 ) ); // 0
Console.WriteLine( Math.Log( 7 ) ); // 1.9459101490553132
Console.WriteLine( Math.Log( 10 ) ); // 2.3025850929940460
Console.WriteLine( Math.Log( 100 ) ); // 4.6051701859880920
Console.WriteLine( Math.Log( 1000 ) ); // 6.9077552789821370
Console.WriteLine( Math.Log( -1 ) ); // NaN
Console.WriteLine( Math.Log( 0 ) ); // -∞ / -Infinity
Console.WriteLine( Math.Log( Double.PositiveInfinity ) ); // ∞ / +Infinity
Console.WriteLine( Math.E ); // 2.718281828459045
// Logarithm with custom base is placed in the below example.
}
}
The Math.Log()
method is presented on the following chart:
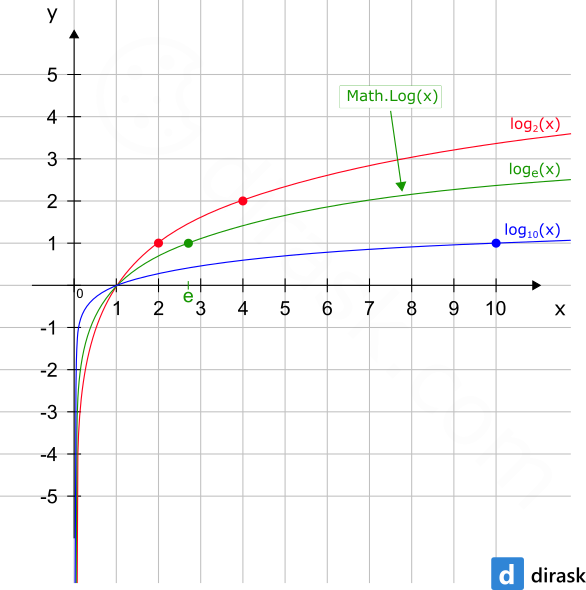
1. Documentation
Syntax |
|
Parameters |
|
Result |
If If If |
Description |
|
2. Logarithm with custom base example
This example shows a logarithmic function calculation with its own base.
using System;
public class Program
{
static double calculateLogarithm(double logBase, double x)
{
double a = Math.Log(x);
double b = Math.Log(logBase);
return a / b;
}
public static void Main(string[] args)
{
// Logarithm with custom base:
// base x y
Console.WriteLine( calculateLogarithm( 2, 2 ) ); // 1
Console.WriteLine( calculateLogarithm( 2, 4 ) ); // 2
Console.WriteLine( calculateLogarithm( Math.E, Math.E ) ); // 1
Console.WriteLine( calculateLogarithm( 3, 9 ) ); // 2
Console.WriteLine( calculateLogarithm( 3, 81 ) ); // 4
Console.WriteLine( calculateLogarithm( 10, 10 ) ); // 1
}
}