EN
JavaScript - sort HTML table by column
12
points
Result of this post:
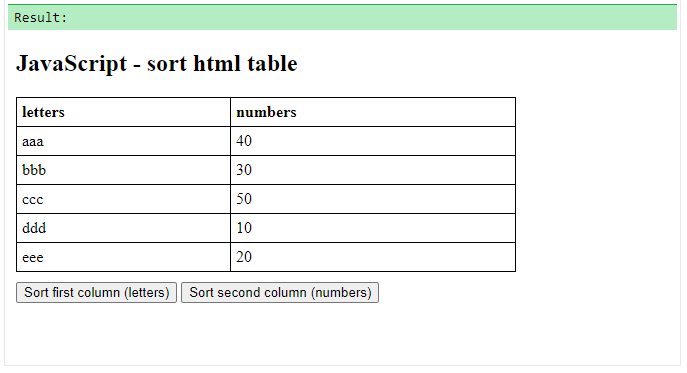
Code:
// ONLINE-RUNNER:browser;
<!doctype html>
<html>
<head>
<meta charset="UTF-8">
<style>
table {
width: 100%;
}
table, th, td {
border: 1px solid black;
border-collapse: collapse;
}
th, td {
padding: 5px;
text-align: left;
}
</style>
</head>
<body style="height: 300px;">
<h2>JavaScript - sort html table</h2>
<table style="width: 500px;">
<tr>
<th>letters</th>
<th>numbers</th>
</tr>
<tr>
<td>ccc</td>
<td>50</td>
</tr>
<tr>
<td>bbb</td>
<td>30</td>
</tr>
<tr>
<td>aaa</td>
<td>40</td>
</tr>
<tr>
<td>eee</td>
<td>20</td>
</tr>
<tr>
<td>ddd</td>
<td>10</td>
</tr>
</table>
<div style="margin-top: 10px;">
<button onclick="sort(0)">Sort first column (letters)</button>
<button onclick="sort(1)">Sort second column (numbers)</button>
</div>
<script>
function sort(columnIndex) {
const table = document.querySelector('table');
const rows = table.rows;
outer:
while (true) {
for (let index = 1; index < rows.length - 1; index++) {
const row1 = rows[index];
const row2 = rows[index + 1];
const text1 = row1.cells[columnIndex].innerText.toLowerCase();
const text2 = row2.cells[columnIndex].innerText.toLowerCase();
if (text1 > text2) {
row1.parentNode.insertBefore(row2, row1);
continue outer;
}
}
break;
}
}
</script>
</body>
</html>