EN
TypeScript - calculate intersection point of two lines for given 4 points
0 points
Intersection point formula for given two points on each line should be calculated in the following way:
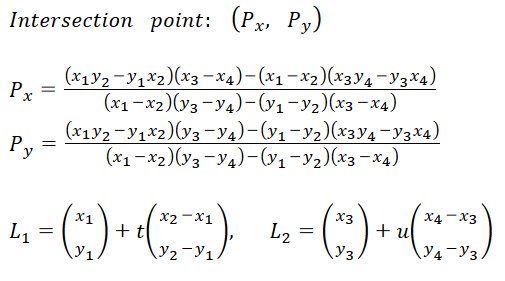
Where:
L1
andL2
represent points on line 1 and line 2 calculated with linear parametric equation. Points(x1, y1)
and(x2, y2)
are located on line 1,(x3, y3)
and(x4, y4)
are located on line 2.
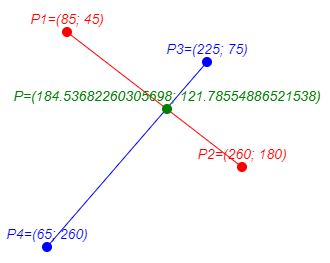
Using TypeScript it is possible to calculate intersection points using the above formula in the following way:
xxxxxxxxxx
1
const calculateIntersection = (
2
p1: Point,
3
p2: Point,
4
p3: Point,
5
p4: Point
6
): Point => {
7
// down part of intersection point formula
8
const d1 = (p1.x - p2.x) * (p3.y - p4.y); // (x1 - x2) * (y3 - y4)
9
const d2 = (p1.y - p2.y) * (p3.x - p4.x); // (y1 - y2) * (x3 - x4)
10
const d = d1 - d2;
11
12
if (d == 0) {
13
throw new Error('Number of intersection points is zero or infinity.');
14
}
15
16
// upper part of intersection point formula
17
const u1 = p1.x * p2.y - p1.y * p2.x; // (x1 * y2 - y1 * x2)
18
const u4 = p3.x * p4.y - p3.y * p4.x; // (x3 * y4 - y3 * x4)
19
20
const u2x = p3.x - p4.x; // (x3 - x4)
21
const u3x = p1.x - p2.x; // (x1 - x2)
22
const u2y = p3.y - p4.y; // (y3 - y4)
23
const u3y = p1.y - p2.y; // (y1 - y2)
24
25
// intersection point formula
26
27
const px = (u1 * u2x - u3x * u4) / d;
28
const py = (u1 * u2y - u3y * u4) / d;
29
30
const p = { x: px, y: py };
31
32
return p;
33
};
34
35
// Usage example:
36
37
interface Point {
38
x: number;
39
y: number;
40
}
41
42
// line 1
43
const p1: Point = { x: 85, y: 45 }; // P1: (x1, y1)
44
const p2: Point = { x: 260, y: 180 }; // P2: (x2, y2)
45
46
// line 2
47
const p3: Point = { x: 225, y: 75 }; // P3: (x4, y4)
48
const p4: Point = { x: 65, y: 260 }; // P4: (x4, y4)
49
50
// result
51
const p: Point = calculateIntersection(p1, p2, p3, p4); // intersection point
52
53
console.log('P=(' + p.x + '; ' + p.y + ')');
Output:
xxxxxxxxxx
1
P=(184.53682260305698; 121.78554886521538)