EN
JavaScript - how to make textarea autogrow?
6 points
In this short article, we are going to look at how to make textarea
element autogrowing according to contained text using JavaScript.
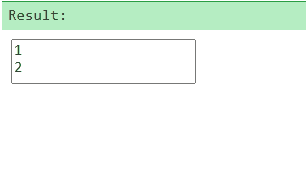
Approach presented in this ection uses following fact: when height
is set as auto
we are able to get scrollHeight
equal to content height that can be used to set as current textarea
height
.
Practical example:
xxxxxxxxxx
1
2
<html>
3
<body>
4
<textarea style="resize: none" id="my-element">Type multi-line text here</textarea>
5
<script>
6
7
function preapreAutogrowing(element) {
8
var style = element.style;
9
function onAction() {
10
style.height = 'auto';
11
style.height = element.scrollHeight + 'px'; // element.scrollHeight should be accessed only when height is set to auto
12
}
13
element.addEventListener('input', onAction);
14
element.addEventListener('change', onAction);
15
var destroyed = false;
16
return {
17
update: onAction,
18
destroy: function() {
19
if (destroyed) {
20
return;
21
}
22
destroyed = true;
23
element.removeEventListener('input', onAction);
24
element.removeEventListener('change', onAction);
25
}
26
};
27
}
28
29
30
// Usage example:
31
32
var element = document.querySelector('#my-element');
33
var autogrowing = preapreAutogrowing(element);
34
35
element.value = '1\n2\n3\n4\n5\n6';
36
37
autogrowing.update(); // forces textarea size update according to contained text
38
// autogrowing.destroy();
39
40
</script>
41
</body>
42
</html>
This approach does not work if word wrapping is enabled.
Note: in different browsers effect can be different (Firefox and Chrome interpret number of rows different way when horisontal scroll is visible)
xxxxxxxxxx
1
2
<html>
3
<head>
4
<style>
5
6
textarea {
7
overflow-y: hidden;
8
white-space: pre;
9
resize: none;
10
}
11
12
</style>
13
<script>
14
15
function preapreAutogrowing(element) {
16
var expression = /\n/g;
17
function onAction() {
18
var text = element.value;
19
if (text) {
20
var match = text.match(expression);
21
if (match) {
22
element.rows = match.length + 2;
23
return;
24
}
25
}
26
element.rows = 2;
27
}
28
element.addEventListener('input', onAction);
29
element.addEventListener('change', onAction);
30
var destroyed = false;
31
return {
32
update: onAction,
33
destroy: function() {
34
if (destroyed) {
35
return;
36
}
37
destroyed = true;
38
element.removeEventListener('input', onAction);
39
element.removeEventListener('change', onAction);
40
}
41
};
42
}
43
44
</script>
45
</head>
46
<body>
47
<textarea id="my-element">Type multi-line text here...</textarea>
48
<script>
49
50
var element = document.querySelector('#my-element');
51
var autogrowing = preapreAutogrowing(element);
52
53
element.value = '1\n2\n3\n4\n5\n6';
54
55
autogrowing.update(); // forces textarea size update according to contained text
56
// autogrowing.destroy();
57
58
</script>
59
</body>
60
</html>