EN
JavaScript - draw line on canvas element?
8 points
In this short article, we would like to show how to draw line on canvas element using JavaScript.
Simple solution:
xxxxxxxxxx
1
// line from x1=10, y1=50 to x2=170, y2=150
2
3
context.beginPath();
4
context.moveTo(10, 50);
5
context.lineTo(170, 150);
6
context.stroke();
Preview:
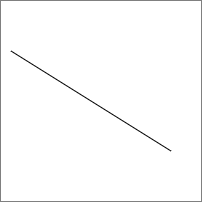
Using JavaScript it is possible to draw line in the following way:
xxxxxxxxxx
1
2
<html>
3
<head>
4
<style>
5
6
#my-canvas { border: 1px solid gray; }
7
8
</style>
9
</head>
10
<body>
11
<canvas id="my-canvas" width="200" height="200"></canvas>
12
<script>
13
14
function drawLine(context, x1, y1, x2, y2) {
15
context.beginPath();
16
context.moveTo(x1, y1);
17
context.lineTo(x2, y2);
18
context.stroke();
19
}
20
21
22
// Usage example:
23
24
var canvas = document.querySelector('#my-canvas');
25
var context = canvas.getContext('2d');
26
27
drawLine(context, 10, 50, 170, 150); // context, x1, y1, x2, y2
28
29
</script>
30
</body>
31
</html>