EN
JavaScript - create style tag
10 points
In Web Browser JavaScript it is possible to create <style>
tag with built-in DOM API.
Quick solution:
xxxxxxxxxx
1
var style = document.createElement('style');
2
3
style.innerText = 'body { background: red; }' +
4
'p { color: blue; }';
5
6
document.head.appendChild(style);
Screenshot:
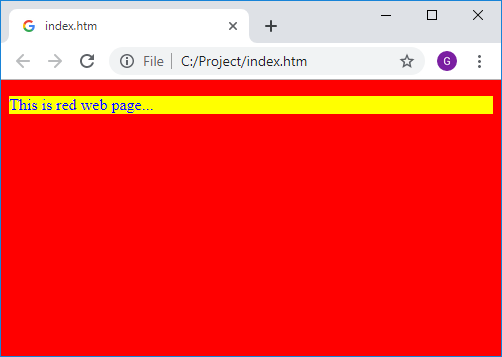
There are different ways how to do it:
In this section, the example shows how to add new CSS rules with text nodes.
xxxxxxxxxx
1
2
<html>
3
<body>
4
<p>This is red web page...</p>
5
<script>
6
7
var element = document.createElement('style');
8
9
element.appendChild(document.createTextNode('body { background: red; }'));
10
element.appendChild(document.createTextNode('body p { background: yellow; color: blue; }'));
11
// appending another rules
12
13
document.head.appendChild(element);
14
15
</script>
16
</body>
17
</html>
It is possible to assign all styles using one assignment of innerText
property.
xxxxxxxxxx
1
2
<html>
3
<body>
4
<p>This is red web page...</p>
5
<script>
6
7
var element = document.createElement('style');
8
9
element.innerText = 'body { background: red; }' +
10
'body p { background: yellow; color: blue; }';
11
12
document.head.appendChild(element);
13
14
</script>
15
</body>
16
</html>