EN
JavaScript - different ways of accessing elements in the DOM
12 points
In this article, we would like to show you how to access elements in the DOM using JavaScript.
xxxxxxxxxx
1
2
<html>
3
<body>
4
<div id="my-tag"></div>
5
<script>
6
7
var handle = document.getElementById('my-tag');
8
9
handle.innerText = 'Hello world!';
10
11
</script>
12
</body>
13
</html>
Result:
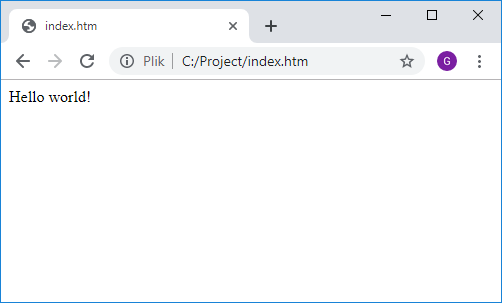
xxxxxxxxxx
1
2
<html>
3
<body>
4
<div>Tag 1</div>
5
<div>Tag 2</div>
6
<div>Tag 3</div>
7
<script>
8
9
var handles = document.getElementsByTagName('div');
10
11
for (var i = 0; i < handles.length; ++i) {
12
handles[i].innerText = 'Hello world!';
13
}
14
15
</script>
16
</body>
17
</html>
Result:
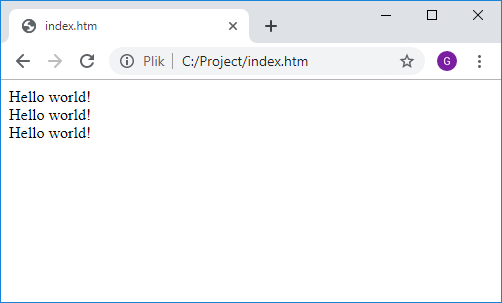
xxxxxxxxxx
1
2
<html>
3
<body>
4
<div class="tag">Tag 1</div>
5
<div class="tag">Tag 2</div>
6
<div class="tag">Tag 3</div>
7
<script>
8
9
var handles = document.getElementsByClassName('tag');
10
11
for (var i = 0; i < handles.length; ++i) {
12
handles[i].innerText = 'Hello world!';
13
}
14
15
</script>
16
</body>
17
</html>
Result:
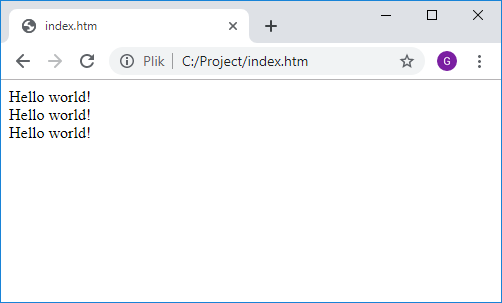
xxxxxxxxxx
1
2
<html>
3
<body>
4
<div id="tag-1" class="tag">Tag 1</div>
5
<div id="tag-2" class="tag">Tag 2</div>
6
<div id="tag-3" class="tag">Tag 3</div>
7
<script>
8
9
var handle = document.querySelector('div'); // gets element handle by tag name
10
11
// Alternatively handle can be get by:
12
// - id value: var handle = document.querySelector('#tag-1');
13
// - class name: var handle = document.querySelector('.tag');
14
// - all other css selectors
15
16
handle.innerText = 'Hello world!';
17
18
</script>
19
</body>
20
</html>
Result:
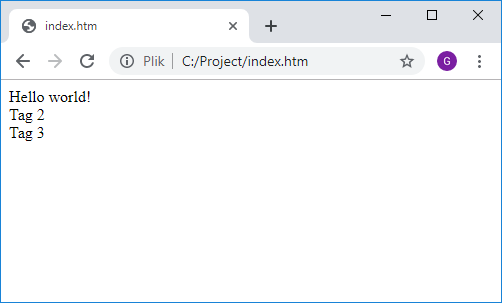
xxxxxxxxxx
1
2
<html>
3
<body>
4
<div id="tag-1" class="tag">Tag 1</div>
5
<div id="tag-2" class="tag">Tag 2</div>
6
<div id="tag-3" class="tag">Tag 3</div>
7
<script>
8
9
var handles = document.querySelectorAll('div'); // gets handles by tag name
10
11
// Alternatively handles can be get by:
12
// - id value: var handles = document.querySelectorAll('#tag-1');
13
// - class name: var handles = document.querySelectorAll('.tag');
14
// - all other css selectors
15
16
for (var i = 0; i < handles.length; ++i) {
17
handles[i].innerText = 'Hello world!';
18
}
19
20
// Warning: id should be unique, so querySelectorAll() with id is not recommended
21
22
</script>
23
</body>
24
</html>
Result:
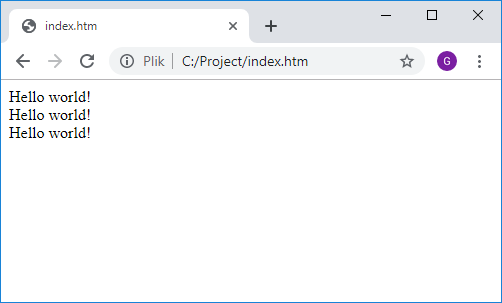
xxxxxxxxxx
1
2
<html lang="en">
3
<head>
4
<meta charset="utf-8">
5
<title>Example</title>
6
<style>
7
8
.block {
9
border: 1px solid red;
10
border-radius: 3px;
11
}
12
13
</style>
14
</head>
15
<body>
16
<h3>Built-in handles example</h3>
17
<script>
18
19
var head = document.head; // html->head handle
20
var body = document.body; // html->body handle
21
var script = document.currentScript; // currently executed script handle
22
23
var container = document.createElement('div');
24
25
function appendText(text) {
26
var handle = document.createElement('pre');
27
handle.className = 'block';
28
handle.innerText = text;
29
container.appendChild(handle);
30
}
31
32
body.style.background = 'blue';
33
34
appendText(head.innerHTML);
35
appendText(script.innerHTML);
36
37
script.parentNode.insertBefore(container, script);
38
39
</script>
40
</body>
41
</html>
Result:
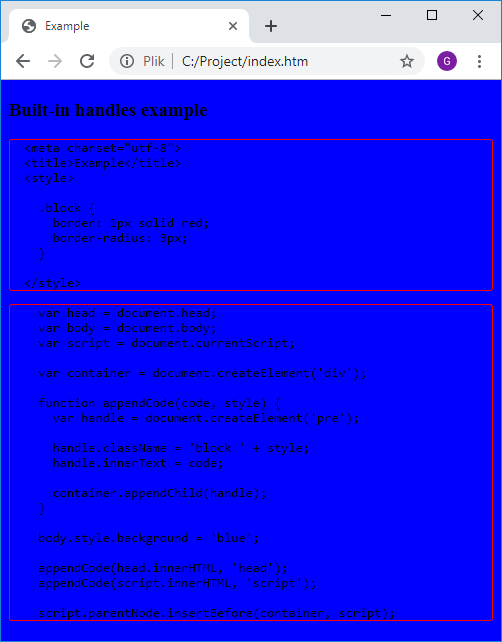