EN
JavaScript - resize event example
0
points
In this article, we would like to show you resize event example in JavaScript.
Quick solution:
var myElement = document.querySelector('#my-element');
myElement.addEventListener('resize', function() {
console.log('resize event occurred.');
});
or:
<div id="my-element" resize="handleResize()">
<!-- div body here... -->
</div>
or:
var myElement = document.querySelector('#my-element');
myElement.onresize = function() {
console.log('onresize event occurred.');
};
Practical examples
There are three common ways how to use resize event:
- with event listener,
- with element attribute,
- with element property.
1. Event listener based example
In this section, we want to show how to use resize
event on window
element via event listener mechanism.
// ONLINE-RUNNER:browser;
<!doctype html>
<html>
<body>
<div>
Window resized: <span id="my-span">0</span> times.
</div>
<script>
var x = 0;
window.addEventListener('resize', handleResize);
function handleResize(){
document.querySelector("#my-span").innerHTML = x += 1;
}
</script>
</body>
</html>
2. Attribute based example
In this section, we want to show how to use resize
event on window
element via attribute.
// ONLINE-RUNNER:browser;
<!doctype html>
<html>
<body onresize="handleResize()">
<div>
Window resized: <span id="my-span">0</span> times.
</div>
<script>
var x = 0;
function handleResize(){
document.querySelector("#my-span").innerHTML = x += 1;
}
</script>
</body>
</html>
3. Property based example
In this section, we want to show how to use resize
event on window
element via property.
// ONLINE-RUNNER:browser;
<!doctype html>
<html>
<body>
<div>
Window resized: <span id="my-span">0</span> times.
</div>
<script>
var x = 0;
window.onresize = function handleResize(){
document.querySelector("#my-span").innerHTML = x += 1;
}
</script>
</body>
</html>
Result
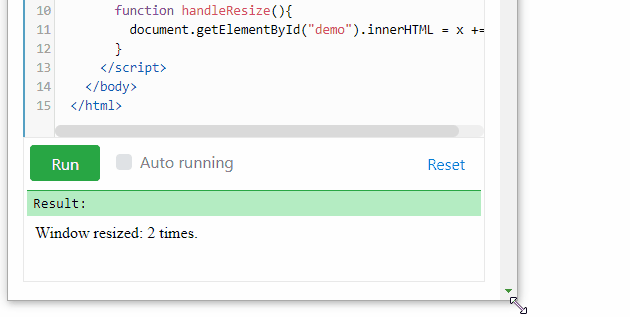