EN
Spring Boot 2 - CRUD example using JPA and MySQL database
3 points
In this article, we would like to show how to use togather: Spring Boot 2, JPA and MySQL database.
Project structure:
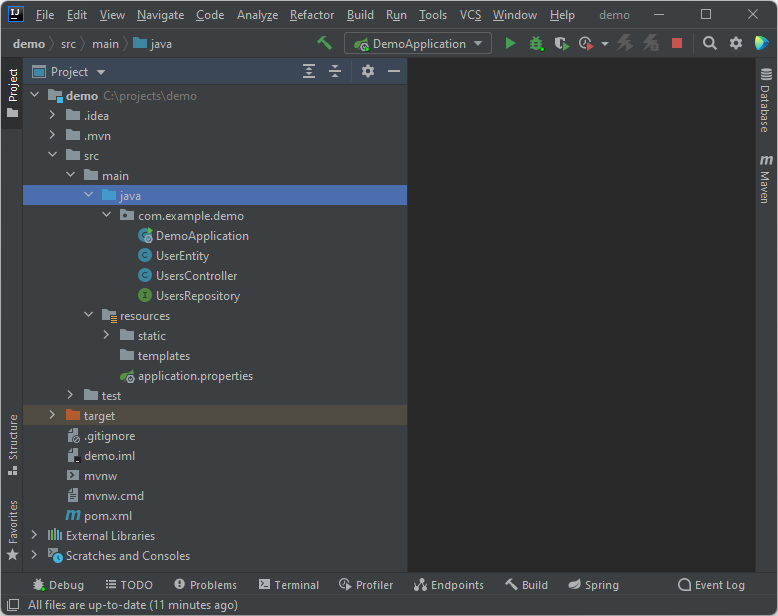
DemoApplication.java
file:
xxxxxxxxxx
1
package com.example.demo;
2
3
import org.springframework.boot.SpringApplication;
4
import org.springframework.boot.autoconfigure.SpringBootApplication;
5
6
7
public class DemoApplication {
8
9
public static void main(String[] args) {
10
SpringApplication.run(DemoApplication.class, args);
11
}
12
}
UsersController.java
file:
xxxxxxxxxx
1
package com.example.demo;
2
3
import org.springframework.beans.factory.annotation.Autowired;
4
import org.springframework.http.MediaType;
5
import org.springframework.http.ResponseEntity;
6
import org.springframework.stereotype.Controller;
7
import org.springframework.transaction.annotation.Transactional;
8
import org.springframework.web.bind.annotation.*;
9
10
import java.util.List;
11
import java.util.Optional;
12
13
14
public class UsersController {
15
16
17
private UsersRepository usersRepository;
18
19
// http://localhost:8080/api/users
20
//
21
(
22
value = "/api/users",
23
produces = MediaType.APPLICATION_JSON_VALUE
24
)
25
readOnly = true) (
26
public ResponseEntity<List<UserEntity>> getUsers() {
27
return ResponseEntity.ok(this.usersRepository.findAll());
28
}
29
30
// http://localhost:8080/api/users/1
31
//
32
(
33
value = "/api/users/{id}",
34
produces = MediaType.APPLICATION_JSON_VALUE
35
)
36
readOnly = true) (
37
public ResponseEntity<UserEntity> getUser( ("id") Long userId) {
38
return ResponseEntity.of(this.usersRepository.findById(userId));
39
}
40
41
// POST http://localhost:8080/api/user/create
42
// echo '{"name":"john","email":"john@email.com"}' | curl -X POST -H "Content-Type: application/json" -d @- http://localhost:8080/api/user/create
43
//
44
(
45
value = "/api/user/create",
46
consumes = MediaType.APPLICATION_JSON_VALUE,
47
produces = MediaType.APPLICATION_JSON_VALUE
48
)
49
50
public ResponseEntity<UserEntity> createUser( UserEntity userEntity) {
51
return ResponseEntity.ok(this.usersRepository.save(userEntity));
52
}
53
54
// POST http://localhost:8080/api/users/1/update
55
// echo '{"name":"chris","email":"chris@email.com"}' | curl -X POST -H "Content-Type: application/json" -d @- http://localhost:8080/api/users/1/update
56
//
57
(
58
value = "/api/users/{id}/update",
59
consumes = MediaType.APPLICATION_JSON_VALUE,
60
produces = MediaType.APPLICATION_JSON_VALUE
61
)
62
63
public ResponseEntity<UserEntity> updateUser( ("id") Long userId, UserEntity newUserEntity) {
64
Optional<UserEntity> foundUserOptional = this.usersRepository.findById(userId);
65
if (foundUserOptional.isPresent()) {
66
UserEntity foundUserEntity = foundUserOptional.get();
67
foundUserEntity.setName(newUserEntity.getName());
68
foundUserEntity.setEmail(newUserEntity.getEmail());
69
this.usersRepository.save(foundUserEntity);
70
}
71
return ResponseEntity.of(foundUserOptional);
72
}
73
74
// http://localhost:8080/api/users/1/remove
75
//
76
(
77
value = "/api/users/{id}/remove",
78
produces = MediaType.APPLICATION_JSON_VALUE
79
)
80
81
public ResponseEntity<UserEntity> removeUser( ("id") Long userId) {
82
Optional<UserEntity> foundUserOptional = this.usersRepository.findById(userId);
83
if (foundUserOptional.isPresent()) {
84
this.usersRepository.deleteById(userId);
85
}
86
return ResponseEntity.of(foundUserOptional);
87
}
88
}
UsersRepository.java
file:
xxxxxxxxxx
1
package com.example.demo;
2
3
import org.springframework.data.jpa.repository.JpaRepository;
4
import org.springframework.stereotype.Repository;
5
6
import java.util.List;
7
import java.util.Optional;
8
9
10
public interface UsersRepository extends JpaRepository<UserEntity, Long> {
11
12
// Hint: use Intellij IDEA Ultimate to get very good intellisense for repositories
13
14
List<UserEntity> findAll();
15
Optional<UserEntity> findById(Long id);
16
17
// See also:
18
//
19
// 1. @Query annotation
20
// 2. Specifications / JpaSpecificationExecutor
21
}
UserEntity.java
file:
xxxxxxxxxx
1
package com.example.demo;
2
3
import javax.persistence.*;
4
5
6
name = "users") (
7
public class UserEntity {
8
9
10
strategy = GenerationType.IDENTITY) (
11
private long id;
12
13
name = "name", nullable = false) (
14
private String name;
15
16
name = "email", nullable = false) (
17
private String email;
18
19
public UserEntity() { }
20
21
public UserEntity(long id, String name, String email) {
22
this.id = id;
23
this.name = name;
24
this.email = email;
25
}
26
27
public long getId() {
28
return this.id;
29
}
30
31
public void setId(long id) {
32
this.id = id;
33
}
34
35
public String getName() {
36
return this.name;
37
}
38
39
public void setName(String name) {
40
this.name = name;
41
}
42
43
public String getEmail() {
44
return this.email;
45
}
46
47
public void setEmail(String email) {
48
this.email = email;
49
}
50
}
application.properties
file:
xxxxxxxxxx
1
db.host=localhost
2
db.port=3306
3
db.name=example_db
4
5
spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver
6
spring.datasource.url=jdbc:mysql://${db.host}:${db.port}/${db.name}?useUnicode=yes&characterEncoding=UTF-8&serverTimezone=UTC&character_set_server=utf8mb4
7
spring.datasource.username=root
8
spring.datasource.password=root
9
10
# May be required by Docker:
11
#
12
# spring.jpa.database-platform=org.hibernate.dialect.MySQLDialect
13
14
# spring.jpa.show-sql=true
15
# spring.jpa.hibernate.ddl-auto=update
Where:
spring.jpa.show-sql=true
enables SQL queries printing in the console,spring.jpa.hibernate.ddl-auto=update
enables automatic tabase preparation when it is needed.
pom.xml
file:
xxxxxxxxxx
1
2
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
3
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
4
5
<modelVersion>4.0.0</modelVersion>
6
7
<parent>
8
<groupId>org.springframework.boot</groupId>
9
<artifactId>spring-boot-starter-parent</artifactId>
10
<version>2.6.5</version>
11
<relativePath/> <!-- lookup parent from repository -->
12
</parent>
13
14
<groupId>com.example</groupId>
15
<artifactId>demo</artifactId>
16
<version>0.0.1-SNAPSHOT</version>
17
<name>demo</name>
18
<description>Demo project for Spring Boot</description>
19
20
<properties>
21
<java.version>1.8</java.version>
22
</properties>
23
24
<dependencies>
25
<dependency>
26
<groupId>org.springframework.boot</groupId>
27
<artifactId>spring-boot-starter-web</artifactId>
28
</dependency>
29
30
<dependency>
31
<groupId>org.springframework.boot</groupId>
32
<artifactId>spring-boot-starter-test</artifactId>
33
<scope>test</scope>
34
</dependency>
35
36
<dependency>
37
<groupId>org.springframework.boot</groupId>
38
<artifactId>spring-boot-starter-data-jpa</artifactId>
39
</dependency>
40
41
<dependency>
42
<groupId>mysql</groupId>
43
<artifactId>mysql-connector-java</artifactId>
44
<version>8.0.22</version>
45
</dependency>
46
</dependencies>
47
48
<build>
49
<plugins>
50
<plugin>
51
<groupId>org.springframework.boot</groupId>
52
<artifactId>spring-boot-maven-plugin</artifactId>
53
</plugin>
54
</plugins>
55
</build>
56
57
</project>
xxxxxxxxxx
1
CREATE DATABASE `example_db`
2
3
4
CREATE TABLE `users` (
5
`id` BIGINT(10) UNSIGNED NOT NULL AUTO_INCREMENT,
6
`name` VARCHAR(100) NOT NULL,
7
`email` VARCHAR(255) NOT NULL,
8
PRIMARY KEY (`id`)
9
)
10
ENGINE=InnoDB;