JavaScript - play web camera on video element (webcam usage example)
In this short article, you can see how to stream video from web camera to video element using JavaScript.
Hint: used solutions were introduced in the major web browsers around 2016.
Quick solution:
// JavaScript:
var video = document.querySelector('#my-video');
var configuration = {
video: true
// you can use microphone adding `audio: true` property here
};
navigator.mediaDevices.getUserMedia(configuration) // Warning: check if getUserMedia() function is avaialble in desired web borwsers
.then(function (stream) {
video.srcObject = stream; // autoplay="true" video attribute starts stream automatically
})
.catch(function (error) {
console.error(error.name + ': ' + error.message);
});
// HTML:
// <video id="my-video" autoplay="true"></video>
Note: it is important to be sure the web camera is connected properly, permissions for camera are granted and it is not used by any other application.
Example preview:
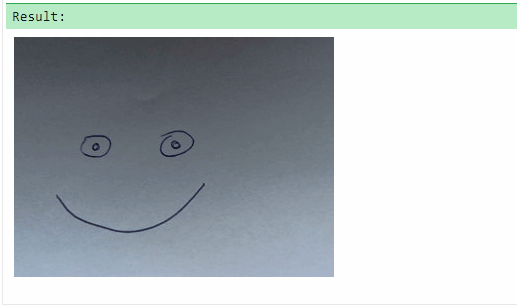
Simple example
In this example, we present how to create reusable logic which can be used to play camera on video HTML element.
Web camera can be run using playCamera()
function that accepts the following agruments:
preferedwidth
- web camera video stream width inpx
(it is just suggestion),preferedheight
- web camera video stream height inpx
(it is just suggestion).
Warning: the logic doesn't provide API to destroy video stream - check next one example.
// ONLINE-RUNNER:browser;
<!doctype html>
<html>
<body>
<video id="my-video"></video>
<script>
function playStream(element, stream) {
var handleLoaded = function() {
element.removeEventListener('loadedmetadata', handleLoaded);
element.play();
};
element.addEventListener('loadedmetadata', handleLoaded);
element.srcObject = stream;
}
function playCamera(element, preferedWidth, preferedHeight) {
var devices = navigator.mediaDevices;
if (devices && 'getUserMedia' in devices) {
var constraints = {
video: {
width: preferedWidth,
height: preferedHeight
}
// you can use microphone adding `audio: true` property here
};
var promise = devices.getUserMedia(constraints);
promise
.then(function(stream) {
playStream(element, stream)
})
.catch(function(error) {
console.error(error.name + ': ' + error.message);
});
} else {
console.error('Camera API is not supported.');
}
}
// Usage example:
var element = document.querySelector('#my-video');
playCamera(element, 640, 480);
</script>
</body>
</html>
Complex example
In this example, we present how to create reusable logic which can be used to play web camera on video HTML element.
In the below example, web camera can be:
- run using
playCamera()
function that accepts the following agruments:preferedwidth
- web camera video stream width inpx
(it is just suggestion),preferedheight
- web camera video stream height inpx
(it is just suggestion),onReady
- function to be executed once the web camera is ready,onError
- function to be executed when some error occurs.
- stopped and destroyed using
stopCamera()
function, e.g.:// ... var stopCamera = playCamera(element, 640, 480, handleReady, handleError); // to stop web camera call: stopCamera();
Source code:
// ONLINE-RUNNER:browser;
<!doctype html>
<html>
<body>
<video id="my-video"></video>
<script>
function playStream(element, stream, callback) {
var handleLoaded = function() {
element.removeEventListener('loadedmetadata', handleLoaded);
element.play();
if (callback) {
callback(stream);
}
};
element.addEventListener('loadedmetadata', handleLoaded);
element.srcObject = stream;
}
function stopStream(stream) {
var tracks = stream.getTracks();
for (var i = 0; i < tracks.length; ++i) {
tracks[i].stop();
}
}
// Plays web camera in indicated video HTML element.
//
function playCamera(element, preferedWidth, preferedHeight, onReady, onError) {
var devices = navigator.mediaDevices;
if (devices && 'getUserMedia' in devices) {
var promise = devices.getUserMedia({
video: {
width: preferedWidth,
height: preferedHeight,
}
// you can use microphone adding `audio: true` property here
});
var _stream = null;
var _destroyed = false;
promise
.then(function(stream) {
if (_destroyed) {
stopStream(stream);
} else {
_stream = stream;
playStream(element, stream, onReady);
}
})
.catch(function(error) {
if (onError) {
onError(error.name + ': ' + error.message);
}
});
// Stops and destroys camera stream.
//
return function() {
if (_destroyed) {
return;
}
if (_stream) {
stopStream(_stream);
_stream = null;
}
_destroyed = true;
};
} else {
if (onError) {
onError('Camera API is not supported.');
}
return function() {
// Nothing here ...
};
}
};
// Usage example:
var element = document.querySelector('#my-video');
var handleReady = function(stream) {
console.log('Web camera is ready and working');
};
var handleError = function(error) {
console.error(error);
};
playCamera(element, 640, 480, handleReady, handleError); // stream [width]x[height] px = 640x480 px
</script>
</body>
</html>
Giving premissions
When a web camera is accessed from JavaScript you should see notification to permit using it. Just let use it by desired website and remove permissions when it is not needed.
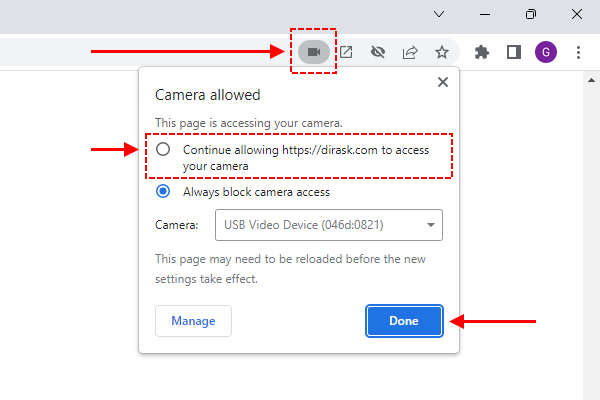