EN
Spring Boot 2 - JdbcTemplate SELECT query example to MySQL database
6 points
In this article, we would like to show how to execute SELECT
query to MySQL database to get items list in Spring Boot 2 application that uses JdbcTemplate
API.
Final result:
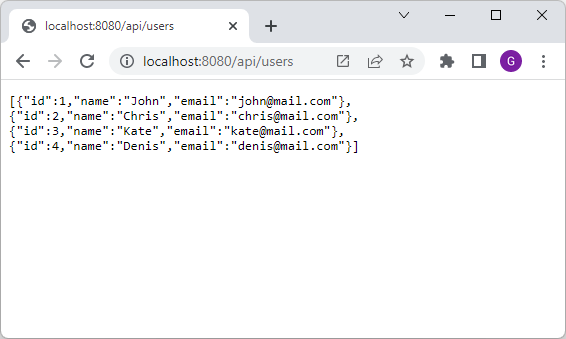
Project structure:
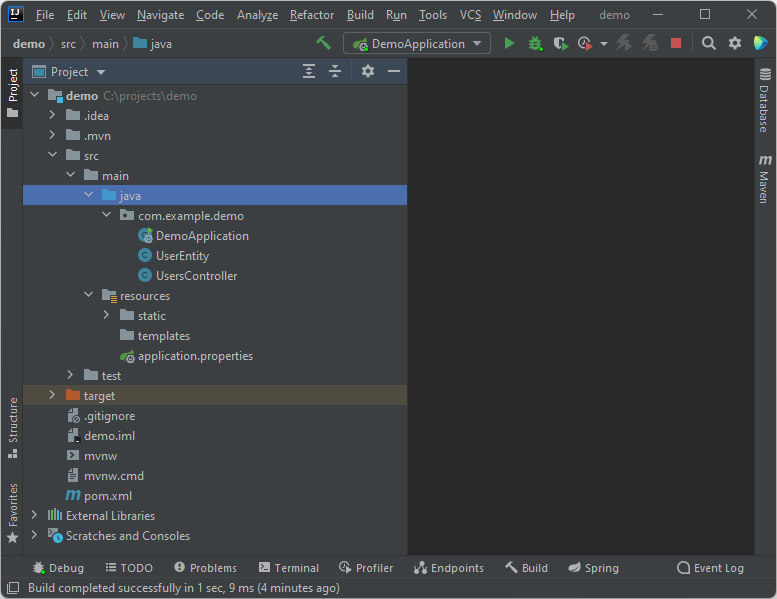
DemoApplication.java
file:
xxxxxxxxxx
1
package com.example.demo;
2
3
import org.springframework.boot.SpringApplication;
4
import org.springframework.boot.autoconfigure.SpringBootApplication;
5
6
7
public class DemoApplication {
8
9
public static void main(String[] args) {
10
SpringApplication.run(DemoApplication.class, args);
11
}
12
}
UsersController.java
file:
xxxxxxxxxx
1
package com.example.demo;
2
3
import org.springframework.beans.factory.annotation.Autowired;
4
import org.springframework.http.MediaType;
5
import org.springframework.jdbc.core.JdbcTemplate;
6
import org.springframework.jdbc.core.RowMapper;
7
import org.springframework.stereotype.Controller;
8
import org.springframework.web.bind.annotation.GetMapping;
9
import org.springframework.web.bind.annotation.ResponseBody;
10
11
import java.sql.ResultSet;
12
import java.sql.SQLException;
13
import java.util.List;
14
15
16
public class UsersController {
17
18
19
private JdbcTemplate jdbcTemplate;
20
21
// http://localhost:8080/api/users
22
//
23
(
24
value = "/api/users",
25
produces = MediaType.APPLICATION_JSON_VALUE
26
)
27
28
public List<UserEntity> getUsers() {
29
String query = "SELECT `id`, `name`, `email` FROM `users`";
30
List<UserEntity> users = this.jdbcTemplate.query(query, new UserRowMapper());
31
return users;
32
}
33
34
private class UserRowMapper implements RowMapper<UserEntity> {
35
36
public UserEntity mapRow(ResultSet resultSet, int rowNumber) throws SQLException {
37
UserEntity user = new UserEntity();
38
user.setId(resultSet.getLong("id"));
39
user.setName(resultSet.getString("name"));
40
user.setEmail(resultSet.getString("email"));
41
return user;
42
}
43
}
44
}
UserEntity.java
file:
xxxxxxxxxx
1
package com.example.demo;
2
3
public class UserEntity {
4
5
private long id;
6
private String name;
7
private String email;
8
9
public UserEntity() { }
10
11
public UserEntity(long id, String name, String email) {
12
this.id = id;
13
this.name = name;
14
this.email = email;
15
}
16
17
public long getId() {
18
return this.id;
19
}
20
21
public void setId(long id) {
22
this.id = id;
23
}
24
25
public String getName() {
26
return this.name;
27
}
28
29
public void setName(String name) {
30
this.name = name;
31
}
32
33
public String getEmail() {
34
return this.email;
35
}
36
37
public void setEmail(String email) {
38
this.email = email;
39
}
40
}
application.properties
file:
xxxxxxxxxx
1
db.host=localhost
2
db.port=3306
3
db.name=example_db
4
5
spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver
6
spring.datasource.url=jdbc:mysql://${db.host}:${db.port}/${db.name}?useUnicode=yes&characterEncoding=UTF-8&serverTimezone=UTC&character_set_server=utf8mb4
7
spring.datasource.username=root
8
spring.datasource.password=root
pom.xml
file:
xxxxxxxxxx
1
2
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
3
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
4
5
<modelVersion>4.0.0</modelVersion>
6
7
<parent>
8
<groupId>org.springframework.boot</groupId>
9
<artifactId>spring-boot-starter-parent</artifactId>
10
<version>2.6.5</version>
11
<relativePath/> <!-- lookup parent from repository -->
12
</parent>
13
14
<groupId>com.example</groupId>
15
<artifactId>demo</artifactId>
16
<version>0.0.1-SNAPSHOT</version>
17
<name>demo</name>
18
<description>Demo project for Spring Boot</description>
19
20
<properties>
21
<java.version>1.8</java.version>
22
</properties>
23
24
<dependencies>
25
<dependency>
26
<groupId>org.springframework.boot</groupId>
27
<artifactId>spring-boot-starter-web</artifactId>
28
</dependency>
29
30
<dependency>
31
<groupId>org.springframework.boot</groupId>
32
<artifactId>spring-boot-starter-test</artifactId>
33
<scope>test</scope>
34
</dependency>
35
36
<dependency>
37
<groupId>org.springframework.boot</groupId>
38
<artifactId>spring-boot-starter-jdbc</artifactId>
39
</dependency>
40
41
<dependency>
42
<groupId>mysql</groupId>
43
<artifactId>mysql-connector-java</artifactId>
44
<version>8.0.22</version>
45
</dependency>
46
47
</dependencies>
48
49
<build>
50
<plugins>
51
<plugin>
52
<groupId>org.springframework.boot</groupId>
53
<artifactId>spring-boot-maven-plugin</artifactId>
54
</plugin>
55
</plugins>
56
</build>
57
58
</project>
xxxxxxxxxx
1
CREATE DATABASE `example_db`
2
3
4
CREATE TABLE `users` (
5
`id` BIGINT(10) UNSIGNED NOT NULL AUTO_INCREMENT,
6
`name` VARCHAR(100) NOT NULL,
7
`email` VARCHAR(255) NOT NULL,
8
PRIMARY KEY (`id`)
9
)
10
ENGINE=InnoDB;
11
12
13
INSERT INTO `users`
14
(`name`, `email`)
15
VALUES
16
('John', 'john@mail.com'),
17
('Chris', 'chris@mail.com'),
18
('Kate', 'kate@mail.com'),
19
('Denis', 'denis@mail.com');