EN
C# / .NET - create multiple subfolders
0 points
In this article, we would like to show you how to create multiple subfolders in C#.
xxxxxxxxxx
1
directory/
2
|
3
+-- subDirectory1/
4
|
5
+-- subDirectory2/
6
|
7
+-- subDirectoryN/
In this example, we use CreateDirectory()
method inside a for loop to create multiple subfolders.
xxxxxxxxxx
1
using System;
2
using System.IO;
3
4
public static class Program
5
{
6
public static void Main(string[] args)
7
{
8
string path = @"C:\directory";
9
10
try
11
{
12
for (int i = 0; i < 3; i++)
13
{
14
string subdirectoryPath = path + "\\subdirectory_" + i;
15
16
if (!Directory.Exists(subdirectoryPath))
17
{
18
DirectoryInfo dir = Directory.CreateDirectory(subdirectoryPath);
19
Console.WriteLine("The directory {0} was created successfully.", subdirectoryPath);
20
}
21
}
22
}
23
catch (Exception e)
24
{
25
Console.WriteLine("The process failed: {0}", e.ToString());
26
}
27
}
28
}
Output:
xxxxxxxxxx
1
The directory C:\directory\subdirectory_0 was created successfully.
2
The directory C:\directory\subdirectory_1 was created successfully.
3
The directory C:\directory\subdirectory_2 was created successfully.
Result:
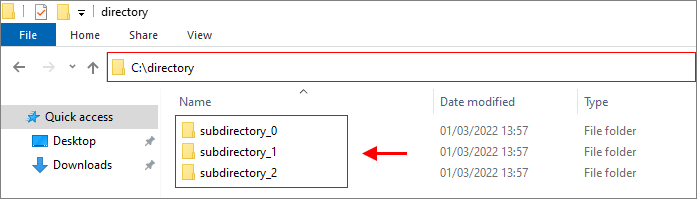