EN
JavaScript - get function argument names
7 points
In this short article we would like to show sinmple way how to get function argument names in JavaScript.
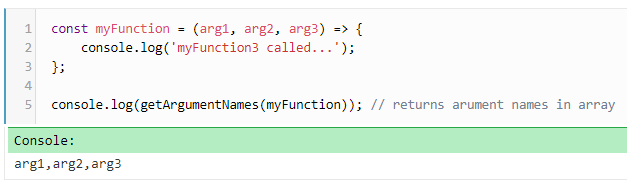
Note: be careful, transpilation and compression may change argument names.
Practical example:
xxxxxxxxxx
1
const functionExpression = /^(?:function(?:\s+[^\(]+)?)?\(\s*([^\)]*\s*)\)/i;
2
const delimiterExpression = /,\s*/i;
3
4
const getArgumentNames = action => {
5
const code = action.toString();
6
const matching = functionExpression.exec(code);
7
if (matching) {
8
const group = matching[1];
9
return group.split(delimiterExpression);
10
}
11
return [];
12
};
13
14
15
// Usage example:
16
17
function myFunction1(arg1) {
18
console.log('myFunction1 called...');
19
}
20
21
const myFunction2 = function FunctionName(arg1, arg2) {
22
console.log('FunctionName called...');
23
};
24
25
const myFunction3 = function(arg1, arg2, arg3) {
26
console.log('myFunction3 called...');
27
};
28
29
const myFunction4 = (arg1, arg2, arg3, arg4) => {
30
console.log('myFunction3 called...');
31
};
32
33
console.log(getArgumentNames(myFunction1));
34
console.log(getArgumentNames(myFunction2));
35
console.log(getArgumentNames(myFunction3));
36
console.log(getArgumentNames(myFunction4));
Note: above example code doesn't wor for code with placed comments inside function declaration (e.g.
function /* note ... */ myFunction() { }
). It is necessary to do some preprocessing to remove comments.