EN
JavaScript - get element by id
2 points
In this article, we would like to show you how to get an element handle by id in JavaScript.
xxxxxxxxxx
1
2
<html>
3
<body>
4
<div id="my-element">Some text here...</div>
5
<script>
6
7
var handle = document.getElementById('my-element');
8
9
handle.style.background = 'red';
10
11
</script>
12
</body>
13
</html>
Result:
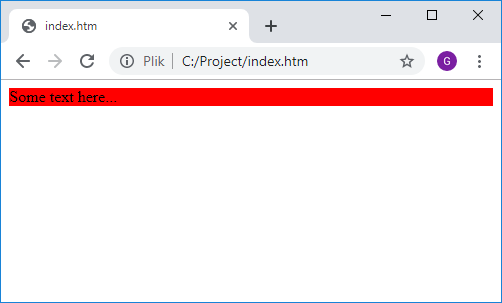
xxxxxxxxxx
1
2
<html>
3
<body>
4
<div id="exampleId">Original text.</div>
5
<button onclick="changeText()">Change Text Button</button>
6
<script>
7
8
var element = document.getElementById('exampleId');
9
10
function changeText() {
11
element.innerHTML = "Changed text.";
12
}
13
14
</script>
15
</body>
16
</html>
xxxxxxxxxx
1
2
<html>
3
<body>
4
<div id="my-element">Some text here...</div>
5
<script>
6
7
var handle = document.querySelector('#my-element');
8
9
handle.style.background = 'red';
10
11
</script>
12
</body>
13
</html>
Result:
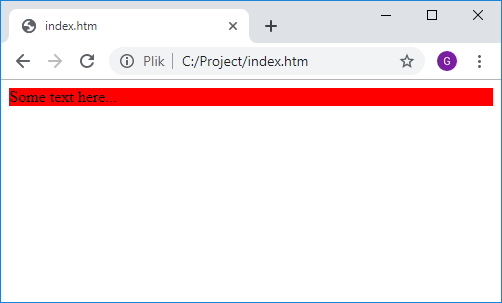
Note:
document.querySelector
method uses css selectors - it means each id name should be prefixed with hash.
Writing web page it is possible to define few elements with same id attribute - this aproach is strongly not recommended because of
id
was designed to be unique.
xxxxxxxxxx
1
2
<html>
3
<body>
4
<div id="my-element">Some text here...</div>
5
<div id="my-element">Some text here...</div>
6
<div id="my-element">Some text here...</div>
7
<script>
8
9
var handles = document.querySelectorAll('#my-element');
10
11
for(var i = 0; i < handles.length; ++i)
12
handles[i].style.background = 'red';
13
14
</script>
15
</body>
16
</html>
Result:
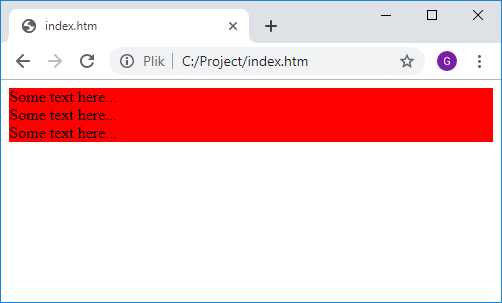