EN
HTML - add css to html
19
points
In this article, we would like to show how to add styles to HTML code (how to add CSS to HTML).
The most popular approaches are: using link
tag, style
tag and style
attribute.
Quick solutions:
1. Link to stylesheet file (*.css
file):
<link rel="stylesheet" type="text/css" href="/path/to/styles.css" />
Hint: put it to
<head>
element.
2. Embedded styles:
<style>
body {
background: red;
}
</style>
Hint: put it somewhere in the source code.
3. Inline styles:
<p style="font-size: 20px; color: brown;">Brown text ...</p>
This article is like an overview of different approaches with examples.
Practical examples
1. Link to stylesheet file example
index.htm
file:
<!doctype html>
<html>
<head>
<link rel="stylesheet" type="text/css" href="styles.css" />
</head>
<body>
Red web page...
</body>
</html>
styles.css
file:
body {
background: red;
}
Result:
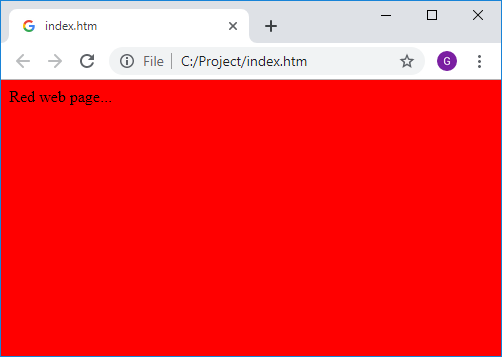
2. Embed CSS (style tag) example
index.htm
file:
// ONLINE-RUNNER:browser;
<!doctype html>
<html>
<head>
<style>
body {
background: red;
}
</style>
</head>
<body>
Red web page...
</body>
</html>
Result:
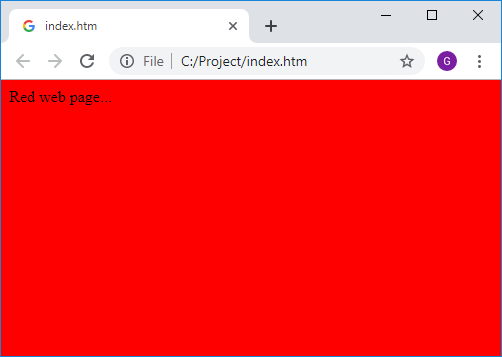
3. Inline styles example
index.htm
file:
// ONLINE-RUNNER:browser;
<!doctype html>
<html>
<body style="background: red;">
<p style="font-size: 20px; color: brown;">
Red web page...
</p>
</body>
</html>
Result:
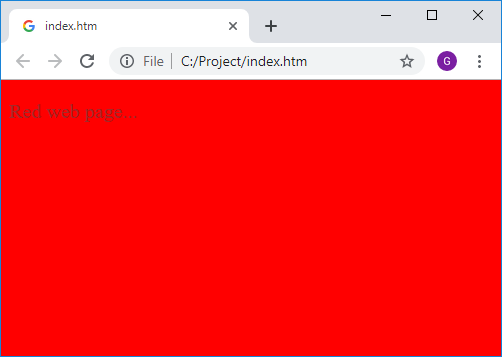
4. Import of stylesheet file example
index.htm
file:
<!doctype html>
<html>
<head>
<style>
@import 'styles.css';
</style>
</head>
<body>
This is red web page...
</body>
</html>
styles.css
file:
body {
background: red;
}
Result:
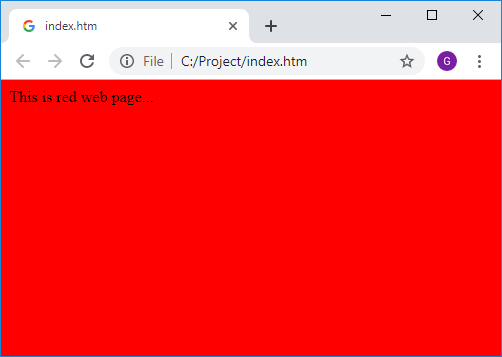
5. JavaScript CSS file injection example
index.htm
file:
<!doctype html>
<html>
<body>
<p>This is red web page...</p>
<script>
var element = document.createElement('link');
element.addEventListener('load', function() {
document.body.innerHTML += '<p>Styles loaded!</p>';
});
element.addEventListener('error', function() {
document.body.innerHTML += '<p>Styles error!</p>';
});
element.setAttribute('rel', 'stylesheet');
element.setAttribute('href', 'styles.css');
document.head.appendChild(element);
</script>
</body>
</html>
styles.css
file:
body {
background: red;
}
Result:
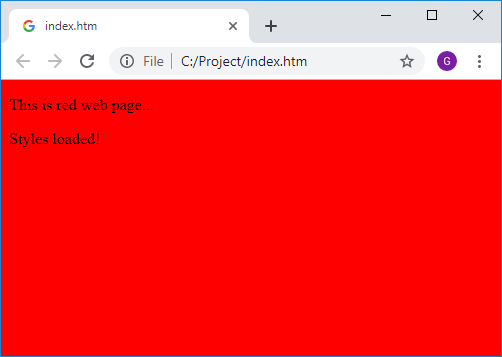
6. JavaScript Embed CSS (style tag) injection example
index.htm
file:
// ONLINE-RUNNER:browser;
<!doctype html>
<html>
<body>
<p>This is red web page...</p>
<script>
var element = document.createElement('style');
element.appendChild(document.createTextNode('body { background: red; }'));
// Add more rules here ...
document.head.appendChild(element);
</script>
</body>
</html>
Result:
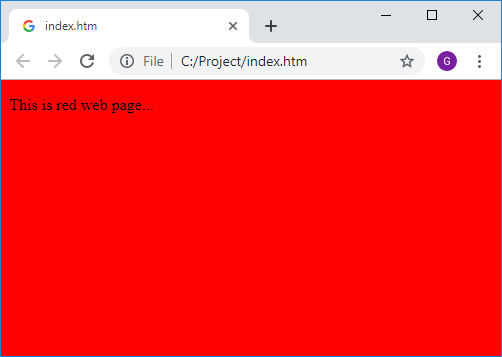
7. JavaScript inline styles example
index.htm
file:
// ONLINE-RUNNER:browser;
<!doctype html>
<html>
<body id="page">
<p>This is red web page...</p>
<script>
var element = document.getElementById('page'); // or document.body
element.style.background = 'red';
element.style.color = 'brown';
//element.style.cssText = 'background: red; color: brown;';
</script>
</body>
</html>
Result:
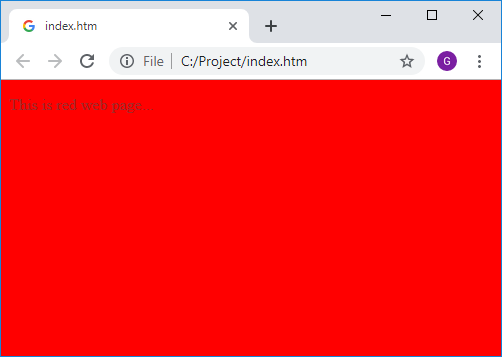
Alternative titles
- HTML - how to attach css to html?
- HTML - how to add css file to html?
- HTML - how to add style file to html?
- HTML - how to attach style file to html?
- HTML - different ways how to attach style to html?
- HTML - different ways how to add style file to html?
- HTML - how to include css to html?
- HTML - how to import css to html?
- HTML - how to import style to html?