Maven skip tests
Most communly used command:
xxxxxxxxxx
mvn clean install -DskipTests=true
# or
# maven.test.skip is honored by Surefire, Failsafe and the Compiler Plugin:
mvn clean install -Dmaven.test.skip=true
In this post we will see how to skip unit tests when we build our project with maven.
Easiest way to skip unit tests with maven is by using one of the commands:
xxxxxxxxxx
mvn clean install -DskipTests=true
# or
mvn install -DskipTests=true
mvn clean install -Dmaven.test.skip=true
# or
mvn install -Dmaven.test.skip=true
or
xxxxxxxxxx
mvn clean package -DskipTests=true
# or
mvn package -DskipTests=true
mvn clean package -Dmaven.test.skip=true
# or
mvn package -Dmaven.test.skip=true
Example of maven clean command:
xxxxxxxxxx
# before running install or package, run clean to clear target directory
mvn clean
Sometimes we may want to build our project without running all unit tests again.
Or some of the unit tests are failing and we want to build the jar anyway.
There is more then one way to accomplish it.
Maven -D parameter means - define a system property, from mvn -help command.
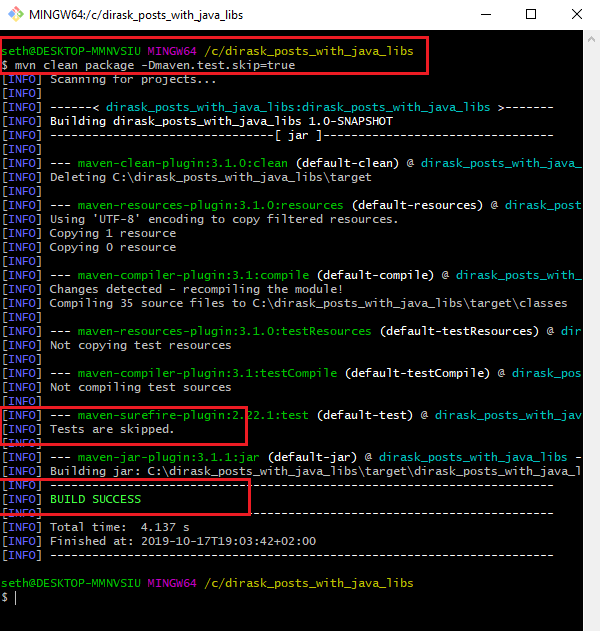
We can run maven commands with defined system property:
xxxxxxxxxx
-Dmaven.test.skip=true
Below we have examples with mvn install and mvn package.
xxxxxxxxxx
mvn install -Dmaven.test.skip=true
# our build will execute without unit tests
xxxxxxxxxx
mvn package -Dmaven.test.skip=true
# our build will execute without unit tests
NOTE: maven.test.skip is honored by:
- Maven Surefire Plugin
- Maven Failsafe Plugin
- Maven Compiler Plugin
Add this property to pom.xml
xxxxxxxxxx
<properties>
<maven.test.skip>true</maven.test.skip>
</properties>
When we have this property defined in our pom.xml, we can run
xxxxxxxxxx
mvn install
# our build will execute without unit tests
or
xxxxxxxxxx
mvn package
# our build will execute without unit tests
Maven will accept property from pom.xml and skip running unit tests.
Add this xml property to Maven Surefire Plugin configuration in pom.xml:
xxxxxxxxxx
<configuration>
<skipTests>true</skipTests>
</configuration>
Full pom.xml example, where we need to add this configuration to skip unit tests:
xxxxxxxxxx
<project>
[...]
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-surefire-plugin</artifactId>
<version>2.20</version>
<!-- HERE it goes -->
<configuration>
<skipTests>true</skipTests>
</configuration>
</plugin>
</plugins>
</build>
[...]
</project>
What does maven -D parameter mean?
It means - define a system property.
xxxxxxxxxx
-D, --define <arg>
To define property we need to use -D.
Synonym of '-D' parameter is '--define'
Examples:
xxxxxxxxxx
mvn install -DskipTests=true
mvn install -DfailIfNoTests=false
When we execture mvn -help, we will get description of all flags.
xxxxxxxxxx
$ mvn -help
..
..
-D, --define <arg> Define a system property
..
..
mvn clean
- will remove our target directory
mvn install
- will build our jar + put it into target dir and copy it to maven .m2 repository on our PC, example of maven local repository path:
xxxxxxxxxx
C:\Users\seth\.m2\repository\com\dirask\0.0.1-SNAPSHOT\dirarsk-examples-0.0.1-SNAPSHOT.jar
mvn package
- will build our jar + put it into target dir, nothing more and nothing less
From maven documentation:
install
- install the package into the local repository, for use as a dependency in other projects locallypackage
- take the compiled code and package it in its distributable format, such as a JAR.
It is good practice to run maven clean command before running mvn install or mvn package command. Maven clean will remove our target directory and in that way we will ensure our code and resources are freshly compiled to target directory.