EN
JavaScript - custom date & time format
6
points
In this article, we would like to show you how to use the custom format date and time in JavaScript.
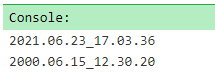
To do this, we will use the padStart
function, which pads the given string with another string until it is the desired length.
Check out the example below to see how it works:
// ONLINE-RUNNER:browser;
const renderNumber = (value, size) => {
const text = String(value);
return text.padStart(size, '0');
};
const formatDate = (date = new Date()) => {
const year = renderNumber(date.getFullYear(), 4);
const month = renderNumber(date.getMonth() + 1, 2);
const day = renderNumber(date.getDate(), 2);
const hours = renderNumber(date.getHours(), 2);
const minutes = renderNumber(date.getMinutes(), 2);
const seconds = renderNumber(date.getSeconds(), 2);
return `${year}.${month}.${day}_${hours}.${minutes}.${seconds}`;
};
// Usage example:
const date = new Date(2000, 05, 15, 12, 30, 20);
console.log(formatDate()); // 2021.03.23_18.37.25
console.log(formatDate(date)); // 2000.06.15_12.30.20
Note:
The
padStart
function is a relatively new feature (ES2017) and may not be compatible with all browsers, it is worth to protect against it and add a polyfill to our web page or paste just following code:
/**
* String.padStart()
* version 1.0.1
* Feature Chrome Firefox Internet Explorer Opera Safari Edge
* Basic support 57 51 (No) 44 10 15
* -------------------------------------------------------------------------------
*/
if (!String.prototype.padStart) {
String.prototype.padStart = function padStart(targetLength, padString) {
targetLength = targetLength >> 0; //floor if number or convert non-number to 0;
padString = String(typeof padString !== 'undefined' ? padString : ' ');
if (this.length > targetLength) {
return String(this);
} else {
targetLength = targetLength - this.length;
if (targetLength > padString.length) {
padString += padString.repeat(targetLength / padString.length); //append to original to ensure we are longer than needed
}
return padString.slice(0, targetLength) + String(this);
}
};
}