Node.js - fs.writeFile() example
In this article, we would like to show you how to use fs.writeFile()
in Node.js.
The fs.writeFile()
method can be used to:
- create new file (if file with the specified name doesn't exist),
- update existing file.
Note:
You can copy each of the examples below and run the script with the following command in the terminal / cmd opened in your project's directory:
node file_name.js
1. Creating new txt file with fs.writeFile()
Below we create file.js
file which creates new txt file in the same directory with some text inside.
Note:
If you want to create file in a different directory just specify relative path to the file as a
fs.writeFile()
first argument instead of just file name.
Practical example:
var fs = require('fs'); // imports File System Module
fs.writeFile('newFile.txt', 'file body here...', (error) => {
if (error) throw error;
console.log('Creating new .txt file');
});
Before:
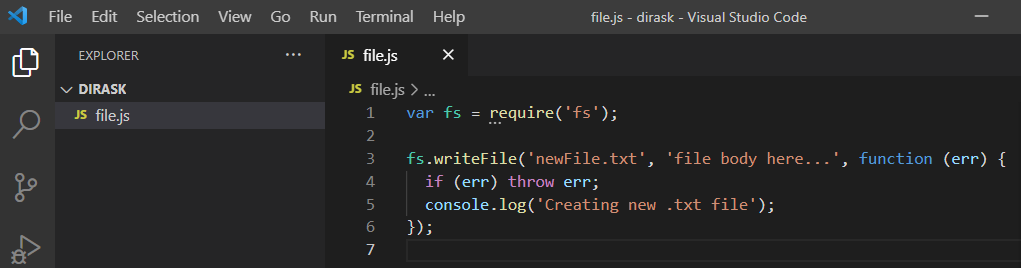
After:
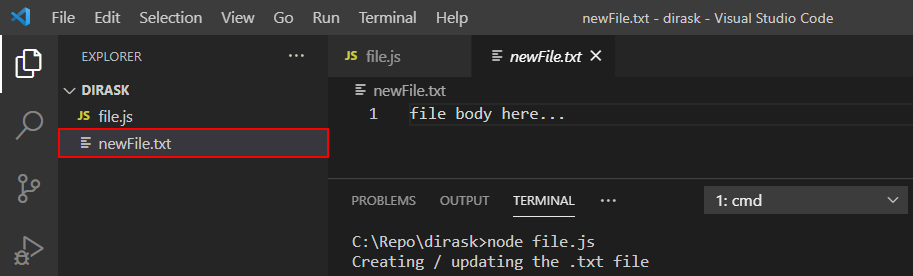
2. Updating txt file with fs.writeFile()
Below we create file.js
file which updates existing txt file in the same directory with some text inside.
Note:
If you want to update file in a different directory just specify relative path to the file as a
fs.writeFile()
first argument instead of just file name.
Practical example:
var fs = require('fs');
var newFileBody = 'new txt file body';
fs.writeFile('newFile.txt', newFileBody, (error) {
if (error) throw error;
console.log('Updating .txt file');
});
Result:
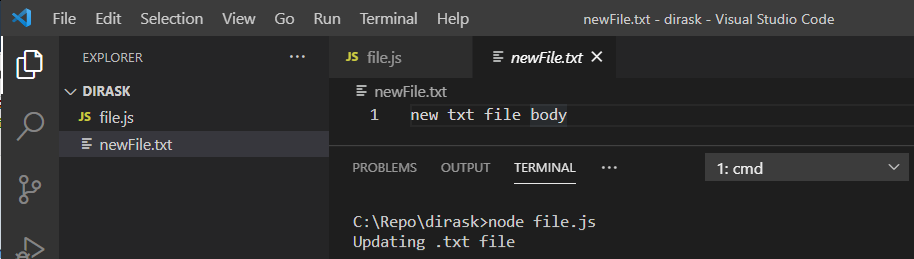
3. fs.write()
example
The fs.write()
method creates a new file with given string or inserts the string at the end of the file if the file exists.
Practical example:
const fs = require('fs');
const str = 'dirask is awesome! \n';
const filename = 'input.txt';
fs.open(filename, 'a', (err, fd) => {
if (err) {
console.log(err.message);
} else {
fs.write(fd, str, (err, bytes) => {
if (err) {
console.log(err.message);
} else {
console.log(bytes + ' bytes written');
}
});
}
fs.close(fd, (err) => {
if (err) console.error('failed to close file', err);
else {
console.log('\n> file closed');
}
});
});
Example result after running script two times:
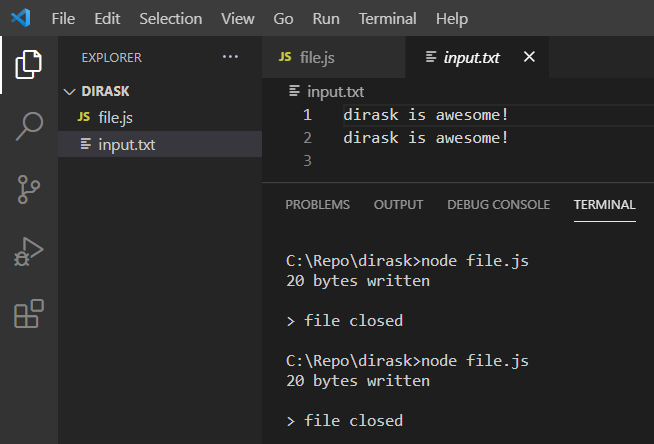