EN
Spring Boot - pre-compile JSP files (Tomcat Server)
8 points
In this short article, we would like to show how to pre-compile *.jsp
files in Spring Boot application that uses Tomcat Server inside.
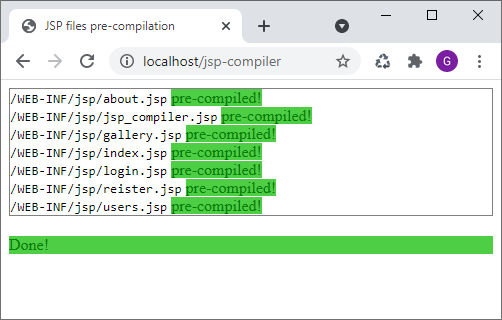
Problem:
*.jsp
files are compiled by Tomcat Server when they are first time used.
Solution: it is necessary to prepare some controller that imports existing
*.jsp
files without executing them - it will force compilation.
The below code shows how to implement JSP files pre-compiler, which should be run always after the Spring Boot Application is started.
Project structure:
xxxxxxxxxx
1
/C/
2
|
3
+-- example-spring-boot-application/
4
|
5
+-- src/
6
| |
7
| +-- main/
8
| |
9
| +-- java/com/example/
10
| | |
11
| | +-- controllers/
12
| | | |
13
| | | +-- JspCompilerController.java
14
| | |
15
| | +-- SpringBootWebApplication.java
16
| |
17
| +-- webapp/WEB-INF/jsp
18
| |
19
| +-- about.jsp
20
| +-- jsp_compiler.jsp
21
| +-- gallery.jsp
22
| +-- index.jsp
23
| +-- login.jsp
24
| +-- reister.jsp
25
| +-- users.jsp
26
|
27
+-- pom.xml
It is necessary to run pre-compilation by opening preapared link:
https://my-domain.com/jsp-compiler
to run pre-compiler on deloyment,http://localhost/jsp-compiler
to run locally developed project if needed.
JspCompilerController.java
file:
xxxxxxxxxx
1
package com.example.controllers;
2
3
import org.springframework.stereotype.Controller;
4
import org.springframework.web.bind.annotation.RequestMapping;
5
import org.springframework.web.bind.annotation.RequestMethod;
6
7
8
public class JspCompilerController {
9
10
value = {"/jsp-compiler"}, method = RequestMethod.GET) (
11
public String getJspCompiler() {
12
13
return "jsp_compiler";
14
}
15
}
jsp_compiler.jsp
file:
xxxxxxxxxx
1
<% page contentType="text/html;charset=UTF-8" language="java" %>
2
<% page import="java.io.IOException" %>
3
<% page import="java.util.Set" %>
4
<% page import="java.util.Iterator" %>
5
<%!
6
private void compileJspFile(
7
ServletContext context,
8
JspWriter output,
9
HttpServletRequest request,
10
HttpServletResponse response,
11
String file
12
) throws IOException {
13
14
output.print("<div class=\"file\"><code>" + file + "</code> ");
15
16
try {
17
RequestDispatcher dispatcher = context.getRequestDispatcher(file);
18
19
if (dispatcher == null) {
20
output.print("<span class=\"error\">not found!</span>");
21
} else {
22
dispatcher.include(request, response);
23
output.print("<span class=\"success\">pre-compiled!</span>");
24
}
25
} catch (Exception ex) {
26
output.print("<span class=\"error\">error!</span>");
27
}
28
29
output.print("</div>");
30
output.flush();
31
}
32
33
private void compileJspFiles(
34
ServletContext context,
35
JspWriter output,
36
HttpServletRequest request,
37
HttpServletResponse response,
38
String directory
39
) throws IOException {
40
41
Set<String> files = context.getResourcePaths(directory);
42
Iterator<String> iterator = files.iterator();
43
44
while (iterator.hasNext()) {
45
String file = iterator.next();
46
47
if (file.endsWith(".jsp")) {
48
compileJspFile(context, output, request, response, file);
49
continue;
50
}
51
52
if (file.endsWith("/")) {
53
compileJspFiles(context, output, request, response, file);
54
continue;
55
}
56
}
57
}
58
%>
59
<html>
60
<head>
61
<title>JSP files pre-compilation</title>
62
<style>
63
64
div.files {
65
border: 1px solid gray;
66
}
67
68
span.error {
69
background: #ce4545;
70
color: #800000;
71
}
72
73
span.success {
74
background: #4ece45;
75
color: green;
76
}
77
78
div.summary {
79
margin: 20px 0 0 0;
80
background: #4ece45;
81
color: green;
82
}
83
84
</style>
85
</head>
86
<body>
87
<div class="files">
88
<%
89
ServletContext context = pageContext.getServletContext();
90
91
HttpServletRequest wrapper = new HttpServletRequestWrapper(request) {
92
public String getQueryString() {
93
/*
94
95
`jsp_precompile` parameter added to URL forces JSP pre-compliation:
96
97
?jsp_precompile
98
?jsp_precompile=true
99
?jsp_precompile=false
100
?some-parameter=here&jsp_precompile=true
101
?some-parameter=here&jsp_precompile=false
102
103
e.g.
104
https://my-domain.com/path/to/api?jsp_precompile=true
105
will force returned JSP view recompliation.
106
107
*/
108
return "jsp_precompile"; // required to run jsp files pre-compilation only
109
};
110
};
111
112
compileJspFiles(context, out, wrapper, response, "/WEB-INF/jsp");
113
%>
114
</div>
115
<div class="summary">Done!</div>
116
</body>
117
</html>