EN
JavaScript - how to make jQuery AJAX POST request with PHP?
2 points
In this article, we would like to show you how to make an AJAX POST request with jQuery to the PHP backend in the following way.
ajax.htm
file:
xxxxxxxxxx
1
2
<html lang="en">
3
<head>
4
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.4.1/jquery.min.js"></script>
5
</head>
6
<body>
7
<pre id="response"></pre>
8
<script>
9
10
var handle = document.getElementById('response');
11
12
$.ajax({
13
type: 'POST',
14
url: '/backend.php',
15
data: {
16
name: 'John',
17
age: 25
18
},
19
success: function (data) {
20
handle.innerHTML = 'Response:\n' + data;
21
},
22
error: function (jqXHR) {
23
handle.innerText = 'Error: ' + jqXHR.status;
24
}
25
});
26
27
</script>
28
</body>
29
</html>
backend.php
file:
xxxxxxxxxx
1
2
3
if ($_SERVER['REQUEST_METHOD'] === 'POST')
4
{
5
echo " Sent data to backend:\n";
6
echo " + Name: " . $_POST['name'] . "\n";
7
echo " + Age: " . $_POST['age'] . "\n";
8
}
9
else
10
{
11
echo " Incorrect request method!";
12
}
Note:
ajax.htm
andbackend.php
files should be placed on php server both.
Result:
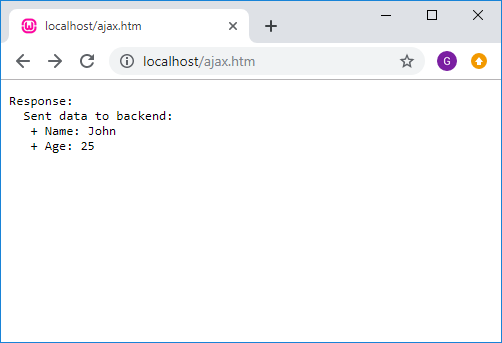
ajax.htm
file:
xxxxxxxxxx
1
2
<html lang="en">
3
<head>
4
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.4.1/jquery.min.js"></script>
5
</head>
6
<body>
7
<pre id="response"></pre>
8
<script>
9
10
var handle = document.getElementById('response');
11
12
var data = {
13
name: 'John',
14
age: 25
15
};
16
17
$.post('/backend.php', data)
18
.done(function (data) {
19
handle.innerHTML = 'Response:\n' + data;
20
})
21
.fail(function (jqXHR) {
22
handle.innerText = 'Error: ' + jqXHR.status;
23
});
24
25
</script>
26
</body>
27
</html>
backend.php
file:
xxxxxxxxxx
1
2
3
if ($_SERVER['REQUEST_METHOD'] === 'POST')
4
{
5
echo " Sent data to backend:\n";
6
echo " + Name: " . $_POST['name'] . "\n";
7
echo " + Age: " . $_POST['age'] . "\n";
8
}
9
else
10
{
11
echo " Incorrect request method!";
12
}
Note:
ajax.htm
andbackend.php
files should be placed on php server both.
Result:
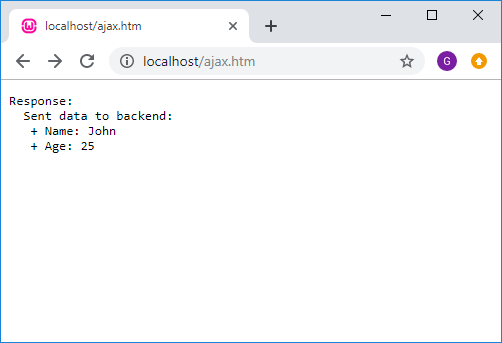