EN
JavaScript - how to redirect to another web page?
8
points
In JavaScript there is available window.location
object that helps to navigate to a web page directly from the font-end level (web browser). location
object provides a few properties and methods from which most useful for making redirection are:
location.replace
methodlocation.assign
methodlocation.href
property- based on the link element (
a
tag withhref
attribute)
For more details look at the below examples.
1. location.replace
method example
<!doctype html>
<html>
<body>
<script>
location.replace('https://dirask.com/about');
</script>
</body>
</html>
Note: this redirection removes current web page from navigation history.
Result:
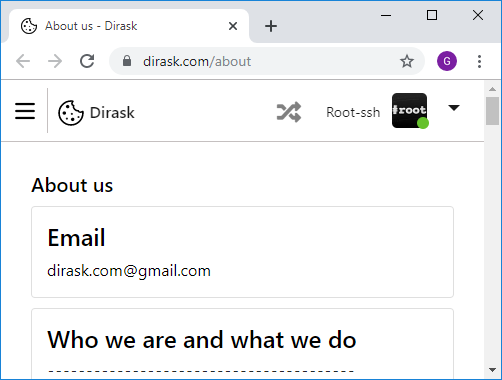
2. location.assign
method example
<!doctype html>
<html>
<body>
<script>
location.assign('https://dirask.com/about');
</script>
</body>
</html>
Note: this redirection keeps current web page in navigation history.
Result:
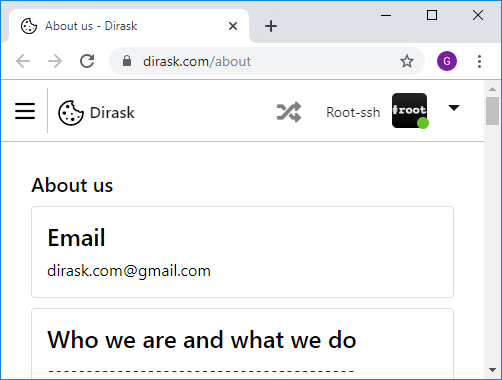
3. location.href
property example
<!doctype html>
<html>
<body>
<script>
location.href = 'https://dirask.com/about';
</script>
</body>
</html>
Note: this redirection keeps current web page in navigation history.
Result:
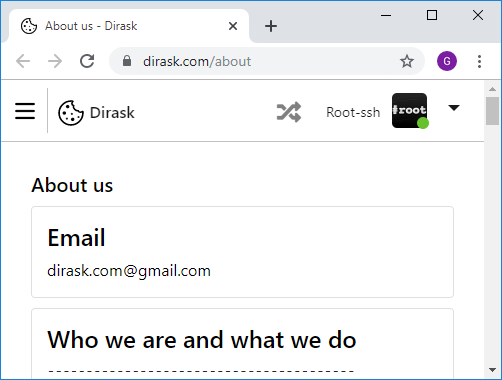
4. Link element example
Note: run it in separated file to see effect
<html>
<body>
<a id="link" href="https://dirask.com"></a>
<script>
var element = document.querySelector('#link');
element.click();
</script>
</body>
</html>