EN
PHP - how to make MySQL delete query with PDO?
5 points
In PHP it is possible to make SQL DELETE
query with PDO in following way.
xxxxxxxxxx
1
2
3
if(isset($_GET['name']))
4
{
5
$name = $_GET['name'];
6
7
$db_name = 'test';
8
$db_host = '127.0.0.1'; // 'localhost'
9
$db_username = 'root';
10
$db_password = 'root';
11
12
$dsn = 'mysql:dbname=' . $db_name . ';host=' . $db_host . ';charset=utf8';
13
$pdo = new PDO($dsn, $db_username, $db_password);
14
15
$statement = $pdo->prepare('DELETE FROM `users` WHERE `name` = :name');
16
17
if($statement === FALSE)
18
die('Query preparation error!');
19
20
$parameters = array(
21
'name' => $name
22
);
23
24
if($statement->execute($parameters))
25
{
26
$count = $statement->rowCount();
27
28
echo 'Number of deleted rows is ' . $count . '.';
29
}
30
else
31
echo 'Query execution error!';
32
}
33
34
Running:
xxxxxxxxxx
1
http://localhost/delete-user.php?name=John
Result:
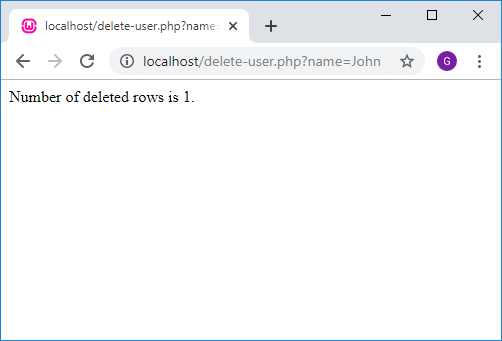
Database:

create_tables.sql
file:
xxxxxxxxxx
1
CREATE TABLE `users` (
2
`id` INT(10) UNSIGNED NOT NULL AUTO_INCREMENT,
3
`name` VARCHAR(100) NOT NULL,
4
`role` VARCHAR(15) NOT NULL,
5
PRIMARY KEY (`id`)
6
)
7
ENGINE=InnoDB;
insert_data.sql
file:
xxxxxxxxxx
1
INSERT INTO `users`
2
(`name`, `role`)
3
VALUES
4
('John', 'admin'),
5
('Chris', 'moderator'),
6
('Kate', 'user'),
7
('Denis', 'moderator');