Spring Boot - change default port 8080 to other port in embedded server
By default Spring Boot application uses embeded tomcat server that starts with default port 8080. Most commons approach to change default port to different is to change application.properties
file configuration.
In this article different technics how to change port In Spring Boot 1 and 2 are described.
In this section same way to configure spring boot port is presented by with different file types.
This solution is dedicated if *.properties
file is used to keep configuration. Add or change line server.port
with own port number.
.../src/main/resources/application.properties
file:
xxxxxxxxxx
server.port=80
or
xxxxxxxxxx
server.port: 80
Note:
=
and:
separators are allowed.
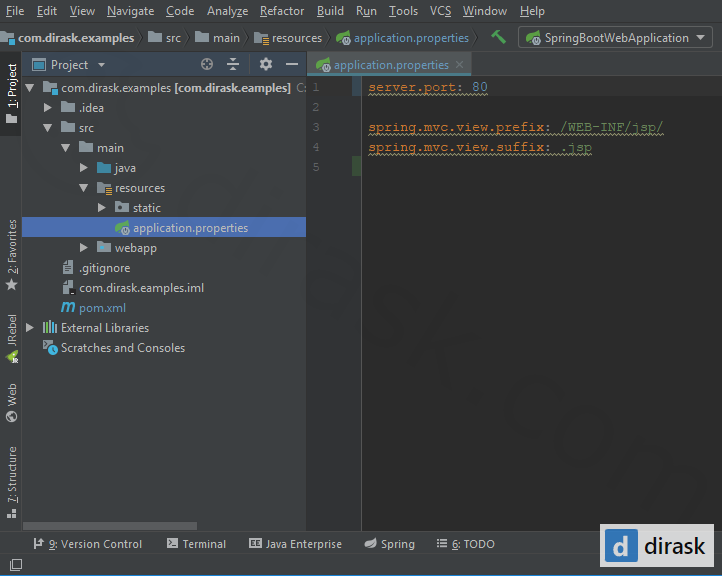
This solution is dedicated if *.yml
file is used to keep configuration. Add server
and port: 80
lines or change line port: 80
with own port number.
.../src/main/resources/application.yml
file:
xxxxxxxxxx
server:
port: 80
Note: do not forget to use indentations in right way.
This approach overides settings located inside properties and yaml from source code level - can be used to override default values too.
Note: read this article to see the exact place where to keep bean configurations.
In this approach EmbeddedServletContainerCustomizer
class is used to create component that overrides configuration.
CustomContainer.java
file:
xxxxxxxxxx
package com.dirask.examples;
import org.springframework.stereotype.Component;
import org.springframework.boot.context.embedded.EmbeddedServletContainerCustomizer;
import org.springframework.boot.context.embedded.ConfigurableEmbeddedServletContainer;
public class CustomContainer implements EmbeddedServletContainerCustomizer {
public void customize(ConfigurableEmbeddedServletContainer container) {
container.setPort(80);
}
}
CustomContainer.java
file location:
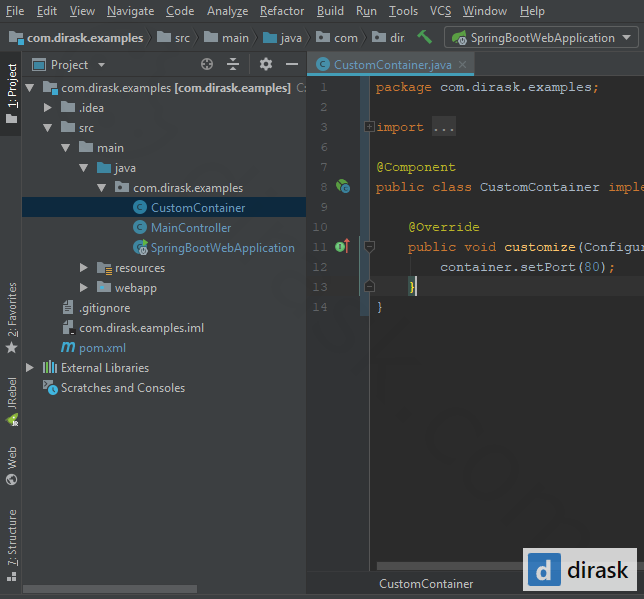
In this approach webServerFactory()
method that returns ConfigurableServletWebServerFactory
object is used.
ServerConfig.java
file:
xxxxxxxxxx
package com.dirask.examples;
import org.springframework.boot.web.embedded.tomcat.TomcatServletWebServerFactory;
import org.springframework.boot.web.servlet.server.ConfigurableServletWebServerFactory;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
public class ServerConfig {
public ConfigurableServletWebServerFactory webServerFactory() {
TomcatServletWebServerFactory factory = new TomcatServletWebServerFactory();
factory.addConnectorCustomizers(connector -> {
connector.setPort(80);
});
return factory;
}
}
ServerConfig.java
file location:
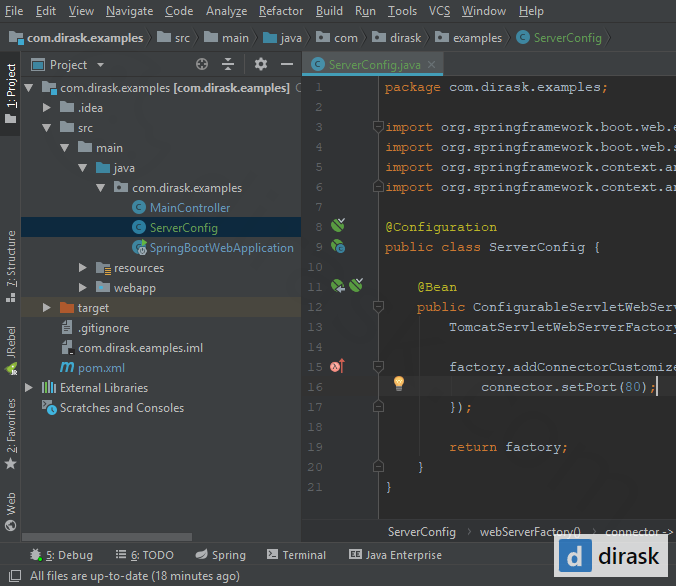
Run your application from command line adding port information.
xxxxxxxxxx
$ java -jar -Dserver.port=80 com.dirask.examples-1.0.jar