EN
VS Code - create Java Maven project
11
points
In this article, we want to show how to create and develop a Java Maven project in VS Code.
Prerequirements
- installed VS Code (link),
- installed Java 11 (JDK) or later (link),
- installed Extension Pack for Java in VS Code (link).
Hints:
Example project
Presented project configuration provides example configuration that uses:
- Lombok preprocessor to generate automatically constructors, getters, setters, etc. in classes,
- JUnit 4 that lets to write unit tests.
Project structure
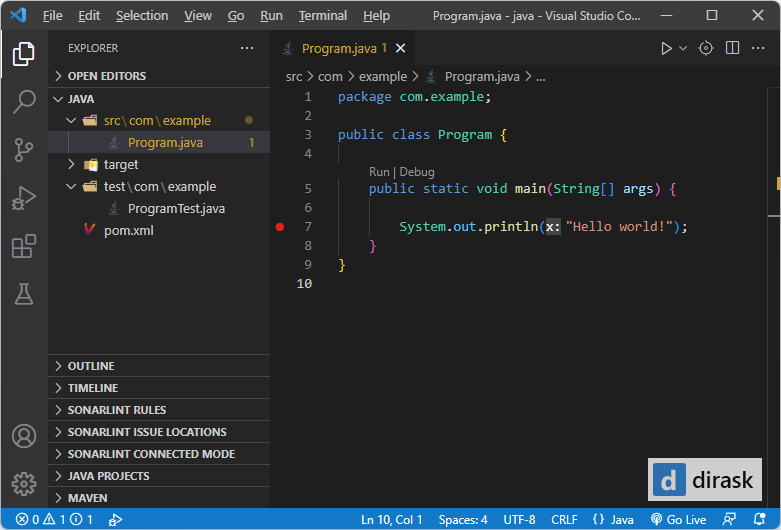
Project preparation
1. Create a directory and go there.
e.g. C:\Projects\my-project
2. Create a Maven project file inside.
e.g. pom.xml
file:
<?xml version="1.0" encoding="UTF-8" standalone="no"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>my-project</artifactId>
<name>My project</name>
<description>My project description.</description>
<version>0.0.1-SNAPSHOT</version>
<packaging>jar</packaging>
<properties>
<java.version>21</java.version>
<maven.compiler.source>${java.version}</maven.compiler.source>
<maven.compiler.target>${java.version}</maven.compiler.target>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding>
</properties>
<dependencies>
<!-- #################### -->
<!-- TESTING -->
<!-- #################### -->
<!-- https://mvnrepository.com/artifact/junit/junit -->
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.13.2</version>
<scope>test</scope>
</dependency>
<!-- https://mvnrepository.com/artifact/org.assertj/assertj-core -->
<dependency>
<groupId>org.assertj</groupId>
<artifactId>assertj-core</artifactId>
<version>3.6.2</version>
<scope>test</scope>
</dependency>
<!-- #################### -->
<!-- PREPROCESSORS -->
<!-- #################### -->
<!-- https://mvnrepository.com/artifact/org.projectlombok/lombok -->
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<version>1.18.34</version> <!-- use latest dependency version -->
<scope>provided</scope>
</dependency>
</dependencies>
<build>
<finalName>${project.artifactId}</finalName>
<sourceDirectory>src</sourceDirectory>
<testSourceDirectory>test</testSourceDirectory>
<plugins>
<plugin>
<!-- https://mvnrepository.com/artifact/org.apache.maven.plugins/maven-compiler-plugin -->
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.8.1</version> <!-- update plugin version if needed -->
<configuration>
<annotationProcessorPaths>
<path>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<version>1.18.34</version> <!-- use latest dependency version -->
</path>
</annotationProcessorPaths>
<source>${maven.compiler.source}</source>
<target>${maven.compiler.target}</target>
<verbose>true</verbose>
</configuration>
</plugin>
<plugin>
<!-- https://mvnrepository.com/artifact/org.apache.maven.plugins/maven-jar-plugin -->
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-jar-plugin</artifactId>
<version>3.4.2</version> <!-- update plugin version if needed -->
<configuration>
<archive>
<manifest>
<mainClass>com.example.Program</mainClass>
</manifest>
</archive>
</configuration>
</plugin>
</plugins>
</build>
</project>
Where:
junit
is optional and allows writing Java tests,assertj-core
is optional and provides nice assertions API,lombok
provides additional Java preprocessor (generates constructors, setters, getters, etc. in classes).
3. Create a Java source code file inside.
e.g. src/com/example/Program.java
file:
package com.example;
public class Program {
public static void main(String[] args) {
System.out.println("Hello world!");
}
}
4. Create a test source code file inside.
e.g. test/com/example/ProgramTest.java
file:
package com.example;
import org.assertj.core.api.Assertions;
import org.junit.Test;
public class ProgramTest {
@Test
public void test() {
String acutal = "Some text here ...";
String expected = "Some text here ...";
Assertions.assertThat(acutal).isEqualTo(expected);
}
// put more tests here ...
}
5. Run in the command line:
mvn clean install
Hint: to skip tests use:
mvn clean install -Dmaven.test.skip=true
6. Open the directory in VS Code.