TypeScript - multiline string
In this article, we're going to have a look at how to create a multiline string in TypeScript.
As multiline string, we mean string that was divided into multiple lines only to be more readable by programmers.
Quick solution:
Since ES6 you can use tempalte literals, so use
`
character instead of'
or"
characters.The character (grave accent) is located togather with Tilde key on the QWERTY keyboard.
let text = `Line 1
Line 2
Line 3`;
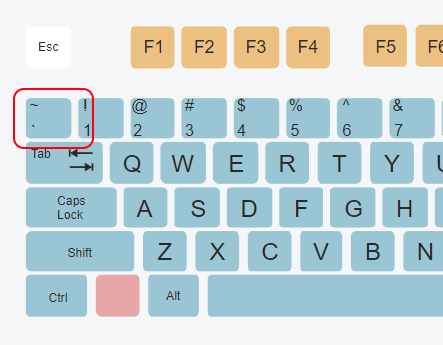
1. Template literals (template strings) example
This approach was introduced in ECMAScript 2015 (ES6) that is supported by TypeScript.
The main disadvantage of this approach is necessary to remove white space prefixes for each line if we want to format string as we use it in the source code.
let text = `Line 1
Line 2
Line 3
Line 4
Line 5`;
console.log(text);
Output:
Line 1
Line 2
Line 3
Line 4
Line 5
2. +
operator with strings example
This approach solves the problem of white character prefixes for formatted source code but introduces some complications about taking cate of "
and +
characters.
let text = 'Line 1\n' +
'Line 2\n' +
'Line 3\n' +
'Line 4\n' +
'Line 5';
console.log(text);
Output:
Line 1
Line 2
Line 3
Line 4
Line 5
3. Backslash at the end of each line example
This approach is very similar to template literals
and introduces problems with taking care of \
character at end of the lines.
Note: this feature is not part of ECMAScript standard, so when source code is transpiled we should be sure it is transpiled to safe code too.
let text = 'Line 1\n\
Line 2\n\
Line 3\n\
Line 4\n\
Line 5';
console.log(text);
Output:
Line 1
Line 2
Line 3
Line 4
Line 5
4. Array
join
method example
This approach is more like a cheat but gives a multiline string effect.
let array: Array<string> = [
'Line 1',
'Line 2',
'Line 3',
'Line 4',
'Line 5'
];
console.log(array.join('\n'));
Output:
Line 1
Line 2
Line 3
Line 4
Line 5