EN
TypeScript - format current date to yyyy-mm-dd (Iso 8601)
0 points
In this article, we would like to show you how to format the current date to yyyy-mm-dd using TypeScript.
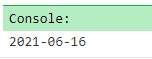
Quick solution:
xxxxxxxxxx
1
// output format: yyyy-mm-dd
2
const getDateInFormat_yyyy_mm_dd = (): string => {
3
const toString = (date: number, padLength: number) => {
4
return date.toString().padStart(padLength, '0');
5
};
6
7
const date = new Date();
8
9
const dateTimeNow = toString(date.getFullYear(), 4) + '-' + toString(date.getMonth() + 1, 2) + '-' + toString(date.getHours(), 2);
10
return dateTimeNow;
11
};
12
13
console.log(getDateInFormat_yyyy_mm_dd()); // 2022-03-13
Output:
xxxxxxxxxx
1
2022-03-13
xxxxxxxxxx
1
// output format: yyyy-mm-dd
2
const getDateInISO_8601 = (): string => {
3
const date = new Date();
4
const year = '' + date.getFullYear();
5
let month = '' + (date.getMonth() + 1);
6
let day = '' + date.getDate();
7
8
if (month.length < 2) {
9
month = '0' + month;
10
}
11
if (day.length < 2) {
12
day = '0' + day;
13
}
14
return year + '-' + month + '-' + day;
15
};
16
17
console.log(getDateInISO_8601()); // 2022-03-14
Output:
xxxxxxxxxx
1
2022-03-14
xxxxxxxxxx
1
const getIso8601Date = (date?: Date): string => {
2
if (date == null) {
3
date = new Date();
4
}
5
6
const year = String(date.getFullYear());
7
let month = String(date.getMonth() + 1);
8
let day = String(date.getDate());
9
10
if (month.length < 2) {
11
month = '0' + month;
12
}
13
if (day.length < 2) {
14
day = '0' + day;
15
}
16
17
return year + '-' + month + '-' + day;
18
};
19
20
21
// Usage example:
22
23
const date = new Date(2021, 5, 13); // 2021-05-13 - e.g. recived from server
24
25
console.log(getIso8601Date(date)); // 2021-06-13
26
console.log(getIso8601Date()); // 2022-03-14
Output:
xxxxxxxxxx
1
2021-06-13
2
2022-03-14