EN
Spring JPA - using entity field names in @Query annotation
4
points
In this post I would like to share that we can use entity field names in @Query annotations when we build new SQL query. Intellij IDEA will help us with intellicence. Entity needs to have getters.
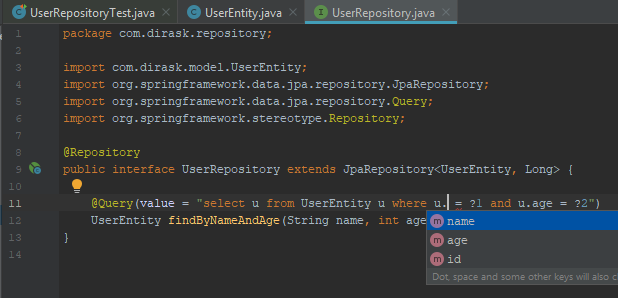
Repository:
package com.dirask.repository;
import com.dirask.model.UserEntity;
import org.springframework.data.jpa.repository.JpaRepository;
import org.springframework.data.jpa.repository.Query;
import org.springframework.stereotype.Repository;
@Repository
public interface UserRepository extends JpaRepository<UserEntity, Long> {
@Query(value = "select u from UserEntity u where u.name = ?1 and u.age = ?2")
UserEntity findByNameAndAge(String name, int age);
}
Entity:
package com.dirask.model;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
import javax.persistence.Table;
@Entity
@Table(name = "users")
public class UserEntity {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private int age;
// constructor, getters, setters
}
Unit test
We can test if it works, download this project and copy repository method + query and run below unit test:
package com.dirask.repository;
import com.dirask.model.UserEntity;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.autoconfigure.orm.jpa.DataJpaTest;
import org.springframework.test.context.junit4.SpringRunner;
import static org.assertj.core.api.Assertions.assertThat;
@RunWith(SpringRunner.class)
@DataJpaTest
public class UserRepositoryTest {
@Autowired
private UserRepository userRepository;
@Test
public void should_find_one_user() {
UserEntity user = userRepository.findByNameAndAge("Kate", 26);
assertThat(user).isNotNull();
// User{id=1, name='Kate', age=26}
System.out.println(user.toString());
}
}
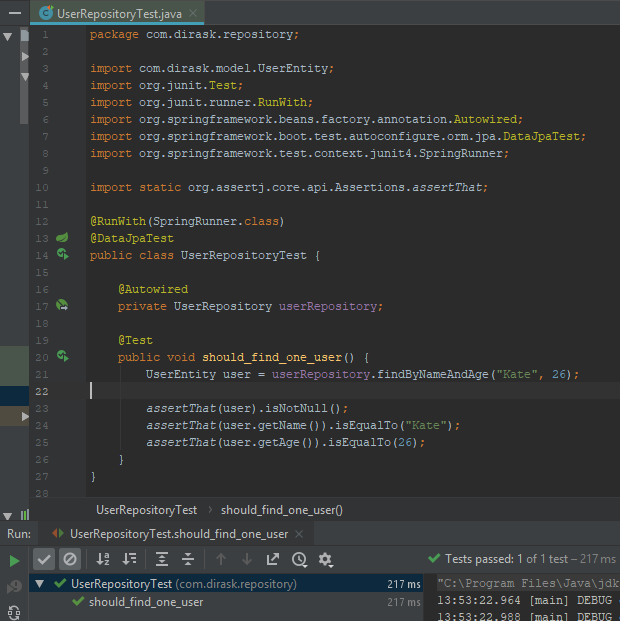
TODO: make repo on github with this exampe based on repo: