PL
React - edytowalna komórka tabeli
0 points
W tym krótkim artykule chcielibyśmy pokazać, jak w technologii React stworzyć komórki, które można edytować po kliknięciu na nie.
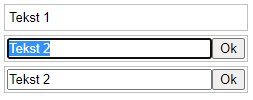
Uwagi:
- Przejdź do tego artykułu (ang), aby dowiedzieć się, jak zmienić stan propsów,
- Przejdź do tego artykułu, aby zobaczyć jak z edytowalnych komórek stworzyć edytowalną tabelę.
Szybkie rozwiązanie:
xxxxxxxxxx
1
// Uwaga: Odkomentuj poniższe linijki podczas pracy z kompilatorem JSX:
2
// import React from 'react';
3
// import ReactDOM from 'react-dom';
4
5
const itemStyle = {
6
padding: '2px',
7
border: '1px solid silver',
8
fontFamily: 'Arial',
9
fontSize: '13px',
10
display: 'flex'
11
};
12
13
const textStyle = {
14
itemStyle,
15
padding: '5px 4px',
16
};
17
18
const inputStyle = {
19
padding: '0',
20
flex: '1',
21
fontFamily: 'Arial',
22
fontSize: '13px'
23
};
24
25
const buttonStyle = {
26
flex: 'none'
27
};
28
29
const Cell = ({value, onChange}) => {
30
const [mode, setMode] = React.useState('read');
31
const [text, setText] = React.useState(value ?? '');
32
React.useEffect(() => {
33
setText(value);
34
}, [value]); // <--- gdy zmieniana jest wartość value, zmienia się również stan
35
if (mode === 'edit') {
36
const handleInputChange = (e) => {
37
setText(e.target.value);
38
};
39
const handleSaveClick = () => {
40
setMode('read');
41
if (onChange) {
42
onChange(text);
43
}
44
};
45
return (
46
<div style={itemStyle}>
47
<input type="text" value={text}
48
style={inputStyle} onChange={handleInputChange} />
49
<button style={buttonStyle} onClick={handleSaveClick}>Ok</button>
50
</div>
51
);
52
}
53
if (mode === 'read') {
54
const handleEditClick = () => {
55
setMode('edit');
56
};
57
return (
58
<div style={textStyle} onClick={handleEditClick}>{text}</div>
59
);
60
}
61
return null;
62
};
63
64
// Przykład:
65
66
const App = () => {
67
const [values, setValues] = React.useState(['Tekst 1', 'Tekst 2', 'Tekst 2']);
68
console.log(values);
69
return (
70
<table style={{width: '250px'}}>
71
<tbody>
72
{values.map((value, index) => {
73
const handleChange = value => {
74
// metoda map() jest używana do aktualizacji wskazanej wartości z kopiowaniem stanu
75
setValues(values.map((v, i) => index === i ? value : v));
76
};
77
return (
78
<tr key={index}>
79
<td><Cell value={value} onChange={handleChange} /></td>
80
</tr>
81
);
82
})}
83
</tbody>
84
</table>
85
);
86
};
87
88
const root = document.querySelector('#root');
89
ReactDOM.render(<App/>, root );